In digital circuits, half adder vs full adder are fundamental components for binary addition. A half adder handles the addition of two bits without considering carry input, while a full adder accounts for carry input along with the two bits. This guide explores their differences and applications in computational design.
Half Adder:
A half adder is a basic digital circuit that performs simple addition of two single-bit binary numbers. It has two inputs: A and B, and two outputs: Sum (S) and Carry (C).
Truth Table:
- A | B | S | C
- 0 | 0 | 0 | 0
- 0 | 1 | 1 | 0
- 1 | 0 | 1 | 0
- 1 | 1 | 0 | 1
Example:
Sum = A XOR B, Carry = A AND B
Advantages:
- Simple design, requires fewer logic gates, easy to implement.
Disadvantages:
- Cannot handle carry from previous addition, limited to adding two bits.
Full Adder:
A full adder is a more complex circuit that adds two single-bit binary numbers along with a carry input. It has three inputs: A, B, and Cin (carry-in), and two outputs: Sum (S) and Carry-out (Cout).
Truth Table:
- A | B | Cin | S | Cout
- 0 | 0 | 0 | 0 | 0
- 0 | 0 | 1 | 1 | 0
- 0 | 1 | 0 | 1 | 0
- 0 | 1 | 1 | 0 | 1
- 1 | 0 | 0 | 1 | 0
- 1 | 0 | 1 | 0 | 1
- 1 | 1 | 0 | 0 | 1
- 1 | 1 | 1 | 1 | 1
Example:
Sum = A XOR B XOR Cin, Cout = (A AND B) OR (B AND Cin) OR (A AND Cin)
Advantages:
- Can handle carry input, suitable for adding multiple bits.
Disadvantages:
- More complex design, requires additional logic gates.
Technical Characteristics:
- Half Adder:
- Logic Equations: Sum = A XOR B, Carry = A AND B
- Inputs: A, B
- Outputs: Sum, Carry
- Full Adder:
- Logic Equations: Sum = A XOR B XOR Cin, Cout = (A AND B) OR (B AND Cin) OR (A AND Cin)
- Inputs: A, B, Cin
- Outputs: Sum, Cout
Use Cases and Applications:
Both half adders and full adders are used in various applications such as:
- Computer processors
- Arithmetic logic units (ALUs)
- Microcontrollers
- Calculator circuits
Key Differences: Half Adder vs Full Adder
Half Adder | Full Adder |
---|---|
Performs addition of two binary bits. | Performs addition of two binary bits along with a carry input. |
Consists of XOR and AND gates only. | Consists of XOR, AND, and OR gates. |
Does not consider any carry from previous additions. | Considers carry from previous additions. |
Sum output is the XOR of the input bits. | Sum output is the XOR of input bits and the carry input. |
Carry output is the AND of the input bits. | Carry output is generated by ANDing the input bits or taking into account the carry input. |
Has 2 inputs and 2 outputs (Sum and Carry). | Has 3 inputs and 2 outputs (Sum and Carry). |
Simple to implement and requires fewer gates. | More complex to implement and requires additional logic gates. |
Used for single-bit addition in simple circuits. | Used in complex arithmetic operations involving multi-bit additions. |
Does not handle carry input from previous calculations. | Handles carry input from previous calculations, enabling cascading for multi-bit addition. |
Less resource-intensive and suited for basic arithmetic in digital electronics. | More resource-intensive due to additional logic gates, suitable for complex arithmetic operations. |
Does not support subtraction operation. | Can be used for subtraction by incorporating 2’s complement method. |
Provides a straightforward solution for simple addition tasks. | Offers a more comprehensive solution for arithmetic operations requiring carry propagation. |
Can be represented with a truth table or logic diagram easily. | Requires a more intricate truth table or logic diagram due to the additional logic gates. |
Forms the basic building block for more complex adders. | Is a component in building higher-order adders and arithmetic units. |
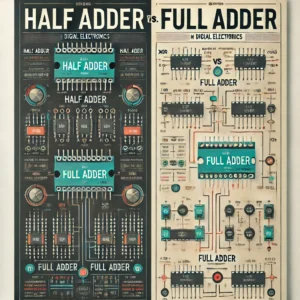
Practical Implementation
Let’s start with a practical example of implementing a half adder and a full adder using logic gates.
Half Adder Implementation
A half adder adds two single-bit binary numbers and generates the sum and carry as outputs.
// Half Adder implementation
function halfAdder(a, b) {
const sum = a ^ b; // XOR gate for sum
const carry = a & b; // AND gate for carry
return { sum, carry };
}
// Example usage
const { sum, carry } = halfAdder(1, 1);
console.log('Sum:', sum, 'Carry:', carry);
Full Adder Implementation
A full adder adds two single-bit binary numbers along with an input carry and generates the sum and carry as outputs.
// Full Adder implementation
function fullAdder(a, b, carryIn) {
const sum = a ^ b ^ carryIn; // XOR gate for sum
const carryOut = (a & b) | (carryIn & (a ^ b)); // OR gate for carry
return { sum, carryOut };
}
// Example usage
const { sum, carryOut } = fullAdder(1, 1, 1);
console.log('Sum:', sum, 'Carry Out:', carryOut);
Step-by-Step Implementation Guide
- Understand the logic gates involved in half adder and full adder.
- Implement the logic for sum and carry using XOR, AND, and OR gates.
- Test the implementations with different input combinations.
Best Practices and Optimization Tips
- Use efficient logic gate configurations to minimize gate count.
- Optimize the code for better performance by reducing unnecessary operations.
- Ensure proper input validation and error handling in the implementations.
Common Pitfalls and Solutions
- Pitfall: Incorrect gate configurations leading to wrong results.
- Solution: Double-check the logic gate connections and truth tables for each operation.
- Pitfall: Missing input carry in the full adder implementation.
- Solution: Include the input carry in the XOR and AND operations to calculate the correct results.
Frequently Asked Questions
What is a Half Adder and how does it differ from a Full Adder?
A Half Adder is a digital circuit that can add two single-bit numbers. It has two inputs, A and B, and two outputs, Sum and Carry. The key difference between a Half Adder and a Full Adder is that a Half Adder does not consider any carry input from previous stages, whereas a Full Adder can handle carry inputs and generate a carry output in addition to the sum.
Can you explain the functionality of a Full Adder in comparison to a Half Adder?
A Full Adder is a more complex digital circuit compared to a Half Adder. It can add two single-bit numbers along with an additional carry input from the previous stage. The Full Adder produces two outputs: Sum and Carry. This allows it to add multiple bits by chaining multiple Full Adders together, enabling the addition of numbers with multiple bits.
What are the key applications of a Half Adder?
A Half Adder is commonly used in simpler digital circuits where only single-bit addition is required. It is suitable for basic arithmetic operations in simple systems, such as in small-scale binary addition tasks or for educational purposes to understand the fundamentals of binary addition.
How is the concept of carry handled differently in Half Adders and Full Adders?
In a Half Adder, there is no provision for handling carry from previous stages. The Half Adder generates a sum output and an immediate carry output if both input bits are 1. In contrast, a Full Adder considers not only the current inputs but also an additional carry input from the previous stage, producing both a sum and a carry output, which enables cascading of adders for multi-bit addition.
When should I use a Half Adder versus a Full Adder in my digital circuit design?
Use a Half Adder when you only need to add two single-bit numbers and do not require handling carry inputs. If your design involves multi-bit addition with carry propagation, opt for a Full Adder or cascaded Full Adders to ensure accurate arithmetic operations. Choosing between a Half Adder and a Full Adder depends on the complexity and requirements of your digital circuit design.
Conclusion
In conclusion, the distinction between a Half Adder and a Full Adder lies in their capabilities to handle carry inputs. While a Half Adder can only add two single-bit numbers without considering any carry from previous operations, a Full Adder can accommodate both the inputs as well as the carry from the previous stage, making it more versatile for complex arithmetic operations.
To make an informed decision on whether to use a Half Adder or a Full Adder, consider the complexity of the arithmetic task at hand. If you are working with simple addition tasks and only need to add two single-bit numbers, a Half Adder would suffice. However, if your calculations involve multi-bit numbers and carry propagation is crucial, opting for a Full Adder would be more appropriate.
Ultimately, the decision-making criteria should be based on the specific requirements of your project, the level of complexity in your arithmetic operations, and the need for carry propagation. By carefully evaluating these factors, you can choose between a Half Adder and a Full Adder to ensure efficient and accurate computation in your digital circuits.