Comparable Interface:
The Comparable interface in Java is used to define a natural ordering of objects. It contains a single method, compareTo(), which is implemented by a class to determine how instances of that class should be ordered.
Example:
class Employee implements Comparable<Employee> {
private String name;
private int id;
public int compareTo(Employee otherEmployee) {
return this.id - otherEmployee.id;
}
}
Comparator Interface:
The Comparator interface in Java is used to define custom ordering of objects. It contains two methods: compare() and equals(). The compare() method is used for comparison logic.
Example:
class EmployeeComparator implements Comparator<Employee> {
public int compare(Employee emp1, Employee emp2) {
return emp1.getName().compareTo(emp2.getName());
}
}
Advantages and Disadvantages
- Comparable:
- Advantages:
- Simple to implement
- Natural order is established
- Disadvantages:
- Cannot be used for custom sorting
- Advantages:
- Comparator:
- Advantages:
- Allows for custom sorting logic
- Can compare objects of different classes
- Disadvantages:
- Requires separate comparator class
- Advantages:
Technical Characteristics
Comparable is an interface defined in the java.lang package, while Comparator is an interface defined in the java.util package. Comparable is used for natural ordering, whereas Comparator is used for custom ordering.
Use Cases and Applications
- Comparable: It is commonly used when the natural ordering of objects needs to be defined, such as sorting a list of strings alphabetically.
- Comparator: It is used when a custom ordering logic needs to be applied, like sorting objects based on multiple attributes or in a specific order.
Key Differences and Analysis
Java Comparable | Java Comparator |
---|---|
Part of the object’s class | Separate external utility |
Can only be implemented by the class being compared | Can be implemented by any class |
Defines the natural ordering of objects | Allows for multiple ways to order objects |
compareTo() method is used for comparison | compare() method is used for comparison |
Single method – compareTo() | Single abstract method – compare() |
Implicitly used by data structures like TreeSet, TreeMap | Explicitly passed to sorting methods like Collections.sort() |
Requires modification of the object’s class | No need to modify the object’s class |
Strongly typed comparison | Flexible comparison logic |
Only one way to sort objects | Multiple ways to sort objects based on different criteria |
Comparable interface is in the java.lang package | Comparator interface is in the java.util package |
Used for natural ordering | Used for customized sorting logic |
Objects are compared based on their natural order | Objects are compared based on a specified order |
Comparable is suitable for objects with a single natural order | Comparator is suitable for objects with multiple sorting criteria |
Comparable interface where T is the type to be compared | Comparator interface where T is the type to be compared |
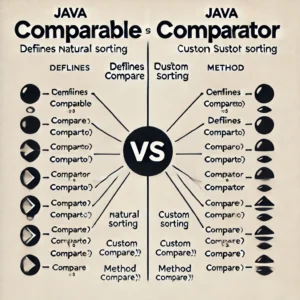
Practical Implementation
Java Comparable and Comparator: Key Differences Explained!
Overview
Java provides two interfaces, `Comparable` and `Comparator`, to facilitate custom sorting of objects. While both interfaces serve the same purpose, they differ in their implementation and use cases.
Comparable Interface:
The `Comparable` interface allows a class to implement a natural ordering of its instances. By implementing the `compareTo()` method, objects can be compared and sorted based on their natural ordering.
Practical Implementation Example:
Suppose we have a `Person` class with attributes `name` and `age`. We can implement the `Comparable` interface to sort `Person` objects based on their age.
public class Person implements Comparable<Person> {
private String name;
private int age;
// Constructor and getters
@Override
public int compareTo(Person otherPerson) {
return Integer.compare(this.age, otherPerson.age);
}
}
Comparator Interface:
The `Comparator` interface allows for custom sorting logic separate from the target class. It is useful when you want to sort objects based on different criteria without modifying the original class.
Practical Implementation Example:
Let’s create a `Comparator` to sort `Person` objects by name.
import java.util.Comparator;
public class PersonNameComparator implements Comparator<Person> {
@Override
public int compare(Person person1, Person person2) {
return person1.getName().compareTo(person2.getName());
}
}
Step-by-Step Implementation Guide:
1. Implement the `Comparable` interface in the target class for natural ordering.
2. Implement the `compareTo()` method in the target class to define the natural ordering logic.
3. Implement the `Comparator` interface in a separate class for custom sorting logic.
4. Implement the `compare()` method in the `Comparator` class to define the custom sorting logic.
Best Practices and Optimization Tips:
- Use `Comparable` for natural ordering and when the sorting logic is intrinsic to the class.
- Use `Comparator` for custom sorting criteria that can change or for sorting third-party classes.
- Implement caching for expensive comparisons to improve performance.
- Consider using lambda expressions for simpler `Comparator` implementations.
Common Pitfalls and Solutions:
Common Pitfall: Forgetting to handle null values in comparisons can lead to `NullPointerExceptions`.
Solution: Always handle null values gracefully in `compareTo()` and `compare()` methods to avoid exceptions.
Implementing the `Comparable` and `Comparator` interfaces in Java allows for flexible and customizable sorting of objects based on different criteria. Understanding the differences and best practices can help in designing efficient sorting mechanisms in your Java applications.
Frequently Asked Questions:
What is the difference between Comparable and Comparator interfaces in Java?
Comparable and Comparator are interfaces in Java used for sorting objects. Comparable is used to define the natural ordering of objects, where the class of the objects being sorted implements the Comparable interface. Comparator, on the other hand, allows for custom sorting logic to be defined separately from the object being sorted.
When should I use Comparable over Comparator?
Use the Comparable interface when you want to define the default or natural ordering of objects within the class itself. Implementing Comparable allows objects of the class to be sorted based on a predefined order. Use Comparator when you need to define multiple sorting criteria or when you want to keep the sorting logic separate from the object’s class.
Can a class implement both Comparable and Comparator interfaces in Java?
Yes, a class can implement both Comparable and Comparator interfaces in Java. Implementing the Comparable interface defines the default sorting order of the objects, while implementing the Comparator interface allows for custom sorting logic to be applied. This flexibility enables developers to sort objects in different ways based on the context.
How does the compareTo() method differ from compare() method in Java?
The compareTo() method is defined in the Comparable interface and is used to compare the current object with another object. It returns a negative integer, zero, or a positive integer as this object is less than, equal to, or greater than the specified object. In contrast, the compare() method is defined in the Comparator interface and is used to compare two objects that are not the objects being sorted.
Which interface is more flexible, Comparable or Comparator?
The Comparator interface is more flexible than Comparable as it allows for defining custom sorting logic that is separate from the object’s class. With Comparator, you can implement different sorting criteria based on specific requirements without modifying the object’s class. This flexibility makes Comparator a preferred choice in scenarios where multiple sorting orders are needed or when sorting logic needs to be changed dynamically.
Conclusion
In conclusion, understanding the differences between Java Comparable and Comparator is essential for effective object comparison and sorting in Java programming.
The key difference lies in the way objects are compared: Comparable is implemented by the class whose instances are being compared, while Comparator allows for external comparison logic to be defined separately.
When deciding between using Comparable or Comparator, consider the following criteria:
1. Object Ownership: If you own the class of objects being compared and can modify their code, implement Comparable. If not, utilize Comparator.
2. Natural Order vs. Custom Order: Use Comparable for the class’s natural ordering, and Comparator for custom ordering that differs from the class’s default ordering.
3. Flexibility and Reusability: Comparator provides more flexibility and reusability by allowing multiple ways to compare objects without modifying their original code.
In general, if you have control over the class code and want to define a default ordering, go for Comparable. If you need multiple sorting options or want to sort objects from external classes, opt for Comparator. By understanding these differences and considering the decision-making criteria, you can efficiently utilize Comparable and Comparator to meet your specific sorting requirements in Java programming.