In object-oriented programming, inheritance vs polymorphism are core principles that improve software design. Inheritance enables one class to acquire the properties of another, promoting code reusability. Polymorphism allows objects to behave differently based on their context, enhancing flexibility. This guide delves into their differences and applications in programming.
Inheritance
Inheritance is a fundamental concept in object-oriented programming that allows a class to inherit attributes and methods from another class. The class that is being inherited from is called the base class or superclass, and the class that inherits from the superclass is called the derived class or subclass.
Example: Consider a Vehicle superclass with attributes like speed and color. A Car subclass can inherit from Vehicle and also have additional attributes like model and brand.
Code Snippet:
class Vehicle: def __init__(self, speed, color): self.speed = speed self.color = color class Car(Vehicle): def __init__(self, speed, color, model, brand): super().__init__(speed, color) self.model = model self.brand = brand
Advantages of Inheritance:
- Code reusability: Inherited classes can reuse the attributes and methods of the superclass.
- Modularity: Inheritance promotes a modular approach to programming by organizing related classes.
Disadvantages of Inheritance:
- Tight coupling: Subclasses are tightly bound to their superclasses, making changes in the superclass affect the subclasses.
- Complexity: Deep hierarchies of inheritance can lead to complex class relationships that are difficult to maintain.
Polymorphism
Polymorphism is the ability of different classes to be treated as instances of a common superclass. It allows objects of different classes to be used interchangeably if they are of a common superclass.
Example: A Shape superclass with subclasses like Circle and Square. Both Circle and Square can be treated as Shapes and have a common method like calculate_area.
Code Snippet:
class Shape: def calculate_area(self): pass class Circle(Shape): def calculate_area(self, radius): return 3.14 radius radius class Square(Shape): def calculate_area(self, side): return side * side
Advantages of Polymorphism:
- Flexibility: Polymorphism allows for dynamic behavior depending on the actual object type at runtime.
- Code extensibility: New subclasses can be added without modifying existing code, promoting scalability.
Disadvantages of Polymorphism:
- Performance overhead: Dynamic dispatch in polymorphism may introduce a slight performance overhead compared to static method calls.
- Complexity: Understanding and managing polymorphic behavior in large codebases can be challenging.
Technical Characteristics:
- Inheritance establishes an “is-a” relationship between classes, while polymorphism enables an “acts-like” relationship.
- Inheritance is a compile-time concept, whereas polymorphism is a runtime concept.
Use Cases and Applications:
- Inheritance: Building class hierarchies for code reuse, modeling real-world relationships, and implementing generic behaviors in superclass.
- Polymorphism: Implementing interfaces in Java, method overriding in C++, and providing different implementations for common behaviors in different subclasses.
Key Differences: Inheritance vs Polymorphism
Inheritance | Polymorphism |
---|---|
Defines a relationship between a base class and derived classes | Allows objects of different classes to be treated as objects of a common superclass |
Supports code reusability by inheriting attributes and methods | Enables flexibility by using a single interface to represent different data types or objects |
Parent-child relationship exists between classes | No parent-child relationship required; objects can be of unrelated types |
Emphasizes an “is-a” relationship between classes | Focuses on behaving differently based on the object’s type |
Derived classes can access and extend the functionality of the base class | Allows for method overriding where the subclass provides a specific implementation for a method defined in the superclass |
Changes in the base class may affect the behavior of derived classes | Behavior of an object can be modified by changing the object it references (dynamic binding) |
Used for code organization and promoting code reuse | Facilitates extensibility and interchangeability of objects at runtime |
Requires the use of keywords like extends (Java) or : (Python) for inheritance | Implementation involves method overloading and method overriding |
Helps in building a hierarchy of classes | Supports multiple forms of polymorphism: compile-time and runtime |
More focused on structure and classification of objects | More focused on behavior and interaction of objects |
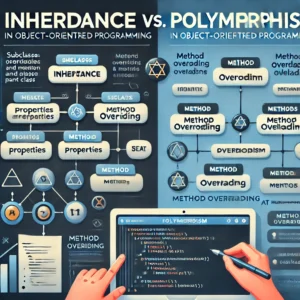
Practical Implementation
Inheritance Example:
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
void sound() {
System.out.println("Dog barks");
}
}
Polymorphism Example:
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
void sound() {
System.out.println("Dog barks");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.sound();
}
}
Step-by-Step Implementation Guide
1. Define a superclass with common properties and methods.
2. Create a subclass that extends the superclass for inheritance.
3. Override methods in the subclass to provide specific implementations.
4. Implement polymorphism by creating objects of subclasses and assigning them to superclass references.
Best Practices and Optimization Tips
- Use inheritance to promote code reuse and establish an “is-a” relationship between classes.
- Prefer composition over inheritance when dealing with complex hierarchies to avoid deep inheritance chains.
- Utilize interfaces to achieve polymorphism in a more flexible and maintainable way.
Common Pitfalls and Solutions
- Pitfall: Overriding methods incorrectly in subclasses can lead to unexpected behavior.
Solution: Ensure method signatures match in subclasses and use @Override annotation for clarity.
- Pitfall: Inappropriate use of inheritance can result in tight coupling and rigid code.
Solution: Favor composition or interfaces to achieve flexibility and reduce dependencies.
By understanding the differences between inheritance and polymorphism, developers can design robust and extensible object-oriented systems efficiently.
Frequently Asked Questions
What is Inheritance in object-oriented programming?
Inheritance is a mechanism in object-oriented programming where a class (called a child or derived class) inherits attributes and methods from another class (called a parent or base class). The child class can reuse and extend the functionality of the parent class.
What is Polymorphism in object-oriented programming?
Polymorphism in object-oriented programming refers to the ability to treat objects from different classes as instances of a common superclass. It allows you to access and manipulate objects from various classes through a single interface, providing flexibility and extensibility in the code.
How do Inheritance and Polymorphism differ?
Inheritance involves creating a new class based on an existing class, allowing the new class to inherit its attributes and behaviors. On the other hand, polymorphism enables objects from different classes to be treated as instances of a common superclass, allowing methods to be invoked dynamically based on the actual type of object at runtime.
Can a class exhibit both Inheritance and Polymorphism simultaneously?
Yes, a class can inherit attributes and methods from a parent class while also exhibiting polymorphic behavior by implementing methods with the same name but different behaviors in its subclasses. This combination enhances code reusability and flexibility.
How are Inheritance and Polymorphism used in real-world applications?
In real-world applications, inheritance models “is-a” relationships, where a subclass represents a specific type of the superclass. Polymorphism allows developers to write more generic and flexible code by enabling different types of objects to be processed uniformly through a shared interface, which enhances code maintainability and scalability.
Conclusion
In conclusion, understanding the distinctions between inheritance and polymorphism is crucial for designing robust and maintainable object-oriented systems. Inheritance allows classes to inherit attributes and behaviors from a parent class, promoting code reusability and establishing an “is-a” relationship. On the other hand, polymorphism enables objects of different classes to be treated as instances of a common superclass, enhancing flexibility and extensibility in software design.
When deciding between inheritance and polymorphism, it is essential to consider the nature of the relationship between classes, the level of code reuse desired, and the potential for future modifications and extensions. If a clear hierarchical relationship exists and code reuse is a priority, inheritance may be the appropriate choice. However, if flexibility and the ability to accommodate various implementations of a common interface are key requirements, polymorphism should be favored.
Ultimately, the decision should be based on the specific needs of the project, weighing factors such as maintainability, extensibility, and the potential impact on code structure. By carefully evaluating these criteria, developers can make informed choices when implementing inheritance and polymorphism in their object-oriented designs.