In Java, HashMap vs HashSet in Java highlights two important collection classes with distinct purposes. HashMap stores key-value pairs, making it useful for fast lookups and data management. In contrast, HashSet stores only unique elements, ensuring no duplicates. Understanding their differences helps in selecting the right data structure for various scenarios.
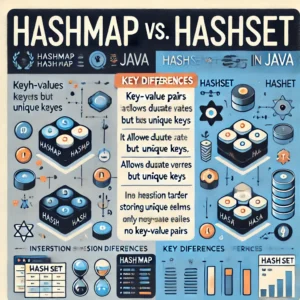
HashMap
HashMap is a data structure that stores elements as key-value pairs. It uses a hash table for storage and allows duplicate values but not duplicate keys. HashMap provides constant-time performance for basic operations like get() and put() operations.
Example:
import java.util.HashMap;
public class HashMapExample {
public static void main(String[] args) {
HashMap<Integer, String> map = new HashMap<>();
map.put(1, "Apple");
map.put(2, "Banana");
map.put(3, "Orange");
System.out.println(map.get(2)); // Output: Banana
}
}
Advantages of HashMap:
- Efficient for key-value pair storage and retrieval
- Allows null key and values
- Supports multithreading
Disadvantages of HashMap:
- Not synchronized, so not thread-safe by default
- Can have performance degradation with a high load factor
HashSet
HashSet is a collection that does not allow duplicate elements. It uses a hash table for storage and provides constant-time performance for basic operations like add(), remove(), and contains().
Example:
import java.util.HashSet;
public class HashSetExample {
public static void main(String[] args) {
HashSet set = new HashSet<>();
set.add("Apple");
set.add("Banana");
set.add("Orange");
System.out.println(set.contains("Banana")); // Output: true
}
}
Advantages of HashSet:
- Efficient for ensuring unique elements
- Allows null elements
Disadvantages of HashSet:
- Not synchronized, so not thread-safe by default
- Cannot store key-value pairs like HashMap
Technical Characteristics
HashMap:
- Stores elements as key-value pairs
- Allows duplicate values but not duplicate keys
- Uses hashing to store and retrieve elements efficiently
HashSet:
- Stores unique elements
- Uses hashing to ensure uniqueness and fast retrieval
Use Cases and Applications
HashMap:
- Storing key-value mappings like database records
- Implementing caches and lookup tables
HashSet:
- Checking for duplicate elements in a collection
- Implementing sets in mathematical applications
Key Differences HashMap vs HashSet
HashMap | HashSet |
---|---|
Implements Map interface, stores key-value pairs | Implements Set interface, stores unique elements |
Uses key to access and retrieve values | Elements are directly accessible |
Allows one null key and multiple null values | Allows only one null element |
Contains methods like put(key, value) and get(key) | Contains methods like add(element) and contains(element) |
Supports iteration over key-value pairs using iterators | Supports iteration over elements using iterators |
Facilitates storing non-unique values with unique keys | Stores only unique elements |
Employs hashing to locate elements efficiently | Also utilizes hashing for quick lookups |
Contains keySet(), values(), and entrySet() methods | Does not contain these specific methods |
Allows key modification (if the hashing of the key is unaffected) | Elements are immutable, keys cannot be modified |
Can have duplicate values with different keys | Ensures all elements are unique |
Overrides equals() and hashCode() for keys | Relies on equals() and hashCode() for elements |
Permits the removal of key-value pairs | Allows removal of elements |
Best suited for key-value mappings and frequent data retrieval | Ideal for storing unique elements and set operations |
Iteration through keySet maintains insertion order | Iteration order is not guaranteed |
Practical Implementation Examples
HashMap Implementation Example
HashMap allows you to store key-value pairs. Here’s a simple Java example:
import java.util.HashMap;
public class HashMapExample {
public static void main(String[] args) {
// Create a new HashMap
HashMap<String, Integer> map = new HashMap<>();
// Add key-value pairs
map.put("apple", 10);
map.put("banana", 5);
// Retrieve values
System.out.println("Value for apple: " + map.get("apple"));
}
}
HashSet Implementation Example
HashSet stores unique elements. Here’s a Java example:
import java.util.HashSet;
public class HashSetExample {
public static void main(String[] args) {
// Create a new HashSet
HashSet<String> set = new HashSet<>();
// Add elements
set.add("apple");
set.add("banana");
set.add("apple"); // Ignored as it's a duplicate
// Iterate over elements
for (String element : set) {
System.out.println(element);
}
}
}
Step-by-Step Implementation Guide
Follow these steps to implement HashMap and HashSet in Java:
- Create a new HashMap or HashSet instance.
- Add elements to the collection using put() for HashMap and add() for HashSet.
- Retrieve or iterate over elements as needed.
Best Practices and Optimization Tips
- Use HashMap when you need to store key-value pairs and HashSet when you need to store unique elements.
- Consider the size of the collection and choose appropriate initial capacities to avoid frequent resizing.
- Use generics to ensure type safety.
Common Pitfalls and Solutions
- Pitfall: Forgetting to override equals() and hashCode() methods when using custom objects as keys in HashMap or elements in HashSet.
- Solution: Always override equals() and hashCode() methods in custom classes to ensure proper functioning of HashMap and HashSet.
Frequently Asked Questions
What is the main difference between HashMap and HashSet?
HashMap is a data structure that stores key-value pairs, while HashSet is a data structure that stores unique elements.
How are HashMap and HashSet implemented internally?
HashMap is implemented using a hashtable, where each key-value pair is stored as an entry in the map. HashSet is implemented using a hashtable as well, but it only stores the unique elements as keys with a dummy value.
Can HashMap and HashSet contain duplicate elements?
No, HashMap cannot contain duplicate keys, but it can have duplicate values associated with different keys. HashSet does not allow duplicate elements.
How do HashMap and HashSet handle null values?
HashMap allows a single null key and multiple null values. HashSet allows a single null element. This means HashSet does not allow duplicate elements, including null values.
Which data structure is more suitable for key-value pair management?
If you need to associate values with unique keys, then HashMap is the better choice. If you only need to store unique elements without any associated values, then HashSet is more appropriate.
Conclusion
In conclusion, the comparison between HashMap and HashSet reveals key distinctions that make each data structure unique. HashMap excels in key-value pair storage and allows for efficient retrieval of values based on keys, offering versatility in handling associations between data elements. On the other hand, HashSet is optimized for maintaining a collection of unique elements, ensuring that no duplicates are stored, which is ideal for scenarios where distinct values are paramount.
When deciding between HashMap and HashSet, it is crucial to consider the nature of the data you are working with and the specific requirements of your application. If your primary concern is efficient searching and retrieval based on keys, HashMap is the preferred choice. Conversely, if you need to store a collection of distinct elements without duplicates, HashSet is more suitable.
The decision-making criteria should revolve around the structure and relationships within your data, the operations you need to perform, and the performance characteristics crucial to your application. By carefully evaluating these factors, you can determine whether HashMap or HashSet is better aligned with your needs and make an informed decision that optimizes the efficiency and effectiveness of your data management.