In Java Streams, map() vs flatMap() are powerful methods for transforming data. While map()
processes each element into a single output, flatMap()
flattens complex structures like nested collections into a streamlined sequence. This guide dives into their differences and practical use cases for efficient data processing.
map()
The map() method is used to transform each element of the stream into another object. It applies a given function to each element and returns a stream of the results. The output stream will have the same number of elements as the input stream.
Example:
List words = List.of("hello", "world");
List wordLengths = words.stream()
.map(String::length)
.collect(Collectors.toList());
// Output: [5, 5]
Advantages of map():
- Simple and straightforward transformation of elements
- Easy to use and understand
Disadvantages of map():
- Not suitable for handling nested collections
flatMap()
The flatMap() method is used to flatten a stream of collections into a single stream of elements. It first maps each element to a stream, then flattens the streams into one stream. This is particularly useful when dealing with nested collections.
Example:
List<List> nestedList = List.of(List.of(1, 2), List.of(3, 4));
List flattenedList = nestedList.stream()
.flatMap(Collection::stream)
.collect(Collectors.toList());
// Output: [1, 2, 3, 4]
Advantages of flatMap():
- Handles nested collections effectively
- Useful for scenarios where each element needs to be mapped to multiple elements
Disadvantages of flatMap():
- Can be complex to understand for beginners
Use Cases and Applications:
map() is commonly used when a one-to-one transformation is required on stream elements, such as converting a list of objects into a list of their properties. On the other hand, flatMap() is ideal for scenarios where a one-to-many transformation is needed, such as flattening nested collections or handling optional values within a stream.
Key Differences: map() vs flatMap()
map() | flatMap() |
---|---|
Operates on each element independently | Can return multiple elements for each input element |
Returns a Stream of elements after applying a function | Returns a single Stream after applying a function that produces another Stream |
Transforms each element into exactly one element | Flattens the result of applying a function, combining all elements into a single Stream |
Does not merge or flatten nested Streams | Can flatten nested Streams by merging their elements into a single Stream |
Useful for one-to-one transformations | Ideal for one-to-many transformations or flattening nested structures |
Preserves the order of elements in the output Stream | May change the order of elements in the output Stream due to merging |
Simple to use and understand for basic transformations | Requires a deeper understanding of Streams and nested structures for effective use |
Applies the function to each element and wraps the result in a Stream | Applies the function to each element, flattening the resulting Streams into one |
Commonly used for transforming data without structural changes | Commonly used for restructuring data and handling complex transformations |
Typically results in a Stream of the same size as the original Stream | May result in a Stream with a different size than the original due to flattening |
Can be used in a chain of operations for sequential transformations | Can complicate the chain due to the potential for nested Streams and order changes |
Less prone to errors related to nested Streams | Requires careful handling to prevent unexpected behavior with nested Streams |
Supports applying functions that return a non-Stream result | Supports applying functions that return a Stream of elements |
Can be a more straightforward choice for basic transformations | Offers more flexibility and power for dealing with complex transformations and nested structures |
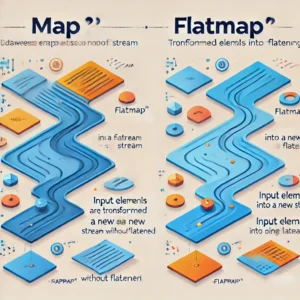
Practical Implementation
Let’s consider a scenario where we have a list of lists and we want to flatten it using both map() and flatMap() operations.
Working code snippets
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<List<Integer>> list = Arrays.asList(
Arrays.asList(1, 2, 3),
Arrays.asList(4, 5, 6),
Arrays.asList(7, 8, 9)
);
List<Integer> flatMappedList = list.stream()
.flatMap(List::stream)
.collect(Collectors.toList());
System.out.println("Flattened List using flatMap: " + flatMappedList);
List<Integer> mappedList = list.stream()
.map(List::stream)
.flatMap(s -> s)
.collect(Collectors.toList());
System.out.println("Flattened List using map: " + mappedList);
}
}
Step-by-step implementation guide
- Create a list of lists containing integers.
- Use flatMap() to flatten the list of lists.
- Use map() and then flatMap() to achieve the same flattening effect.
- Collect the results using Collectors.toList().
Best practices and optimization tips
- Prefer flatMap() over map() when flattening nested collections.
- Use flatMap() when you have a one-to-many mapping.
- Optimize performance by avoiding unnecessary intermediate operations.
Common pitfalls and solutions
One common pitfall when using map() is that it creates a Stream of Streams, which requires an additional flatMap() operation to flatten the result. To avoid this, use flatMap() directly to flatten nested collections.
Frequently Asked Questions
What is the difference between map() and flatMap() in Java Streams?
map() and flatMap() are both methods in Java Streams used for transforming elements. The key difference is that map() transforms each element into another single element, while flatMap() can transform each element into zero or more elements and flattens the result into a single stream.
When should I use map() in Java Streams?
Use map() when you want to transform each element in a stream to exactly one element. This is useful for scenarios where you need a one-to-one mapping of elements, such as converting objects to a different type or extracting specific properties from objects.
Why would I choose flatMap() over map() in Java Streams?
You should choose flatMap() when the transformation of each element results in multiple elements, or when you need to flatten a stream of streams into a single stream. This is commonly used when dealing with nested collections or when you want to expand or collapse elements in a stream.
Can map() and flatMap() be combined in Java Streams?
Yes, map() and flatMap() can be combined in Java Streams to perform complex transformations. You can use map() to transform each element and then use flatMap() to further process the elements if needed, allowing for flexible data manipulation within a stream pipeline.
Are there performance considerations when choosing between map() and flatMap() in Java Streams?
There can be performance implications when using flatMap() compared to map() due to the flattening of nested streams. If the transformation using flatMap() involves costly operations, it may impact performance. It is important to consider the structure of your data and the desired output when deciding between map() and flatMap().
Conclusion
In conclusion, understanding the distinctions between map() and flatMap() in Java Streams is essential for harnessing the full power and flexibility of stream processing. While map() transforms each element of a stream independently, flatMap() allows for transforming each element into multiple elements or even into a completely different stream.
Based on the context and requirements of your project, the decision between map() and flatMap() should be guided by specific criteria. Use map() when you need to apply a one-to-one transformation to the elements in the stream. On the other hand, opt for flatMap() when you want to flatten nested collections, transform each element into multiple elements, or deal with optional values.
In summary, by carefully assessing your transformation needs and the structure of your data, you can make informed decisions between map() and flatMap() to effectively manipulate Java Streams and unlock the magic of stream processing in your applications.