In the world of C programming, understanding the nuances between Call by Value and Call by Reference is crucial for writing efficient and effective code. Let’s delve into this comparison from a fresh perspective to uncover why it matters in practice.
Call by Value
When a function is called by value in C, a copy of the actual parameter is passed to the function. This means any modifications made to the parameter inside the function do not affect the original value outside the function.
Imagine you are ordering a pizza. Call by value is akin to placing an order for a specific pizza without altering the original recipe. You get your customized pizza, but the original recipe remains untouched.
Advantages:
- Prevents unintended modifications to original data
- Simplifies debugging by isolating changes within functions
Disadvantages:
- Requires additional memory for copying data
- Can be less efficient for large data structures
Example:
#include
void increment(int num) {
num++;
}
int main() {
int value = 5;
increment(value);
printf("Value after increment: %d\n", value);
return 0;
}
Call by Reference
In contrast, call by reference passes the address of the actual parameter to the function. This allows modifications inside the function to directly affect the original value outside the function.
Think of call by reference as sharing a recipe with someone. Changes made to the shared recipe impact everyone using it, just like modifications to a reference parameter affect the original value.
Advantages:
- Enables functions to modify original data directly
- Reduces memory consumption by avoiding data duplication
Disadvantages:
- Complex to track changes across functions
- Potential for unintended side effects on shared data
Example:
#include void increment(int *num) { (*num)++; } int main() { int value = 5; increment(&value); printf("Value after increment: %d\n", value); return 0; }
Key Differences between Call by Value and Call by Reference
Call by Value | Call by Reference |
---|---|
Creates a copy of the argument passed to the function | Receives the memory address of the argument |
Changes made to the parameter inside the function do not affect the original argument | Changes made to the parameter inside the function reflect in the original argument |
Requires less memory overhead as it only deals with values | May consume more memory as it involves passing memory addresses |
Arguments are passed by value unless explicitly specified | Arguments are passed by reference unless explicitly specified |
Can be a safer option as it prevents unintended side effects on the original data | Can improve performance in cases where large data structures need to be passed |
Cannot modify the original data passed from the calling function | Allows for direct modification of the original data passed from the calling function |
Efficient for simple data types like integers and characters | Useful when modifications to the original data are desired |
Does not require the use of pointers | Often involves the use of pointers to reference the original data |
Arguments are isolated within the function scope | Allows for sharing and updating data across multiple functions |
Changes made to parameters do not reflect outside the function | Changes made to parameters persist beyond the function call |
Commonly used in simple functions with basic data manipulation | Preferred in situations where the original data needs to be altered |
Useful for maintaining data integrity by preventing unintentional changes | Offers flexibility in managing complex data structures and objects |
Suitable for functions that do not require updating the original data | Beneficial when working with large datasets or complex data structures |
Affects only the local copy of the argument passed | Affects the actual data passed from the caller |
In-Depth Analysis:
When deciding between call by value and call by reference, consider the trade-offs. Call by value offers safety and simplicity but incurs memory overhead, while call by reference provides efficiency but requires careful management to avoid unintended consequences.
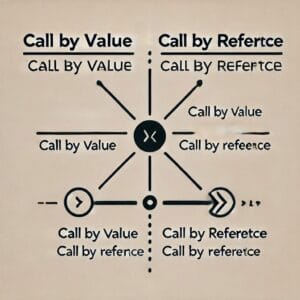
Practical Implementation
Call by Value
In call by value, a copy of the actual parameter is passed to the function.
#include <stdio.h>
void changeValue(int x) {
x = 10;
}
int main() {
int num = 5;
changeValue(num);
printf("Value after function call: %d\n", num);
return 0;
}
Call by Reference
In call by reference, the address of the actual parameter is passed to the function.
#include <stdio.h>
void changeValue(int *x) {
*x = 10;
}
int main() {
int num = 5;
changeValue(&num);
printf("Value after function call: %d\n", num);
return 0;
}
Step-by-Step Implementation Guide
- Create a function that takes an argument by value or by reference.
- In the main function, declare a variable and pass it to the function.
- Observe the behavior of the variable after the function call.
Best Practices and Optimization Tips
- Use call by value for simple data types to avoid unintended side effects on the original variable.
- Use call by reference for complex data structures to avoid the overhead of copying large amounts of data.
- Ensure proper memory management when using call by reference to prevent memory leaks.
Common Pitfalls and Solutions
One common pitfall is mistakenly using call by value when call by reference is required for modifying the original variable.
Solution: Understand the requirements of your program and choose the appropriate method of parameter passing.
Frequently Asked Questions
What is Call by Value in C Programming?
Call by Value is a method used in C programming where the actual value of an argument is passed to a function. In Call by Value, a copy of the argument’s value is passed to the function, and any changes made to the parameter inside the function do not affect the original value outside the function.
What is Call by Reference in C Programming?
Call by Reference is a method used in C programming where the address of the argument is passed to a function. In Call by Reference, changes made to the parameter inside the function directly affect the original value outside the function because the function operates on the actual memory location.
How does Call by Value work in C Programming?
In Call by Value, a copy of the argument’s value is passed to the function, and the function works on this copy. Any modifications made to the parameter inside the function do not reflect back to the original value passed as an argument. Call by Value is useful when you want to prevent unintended changes to the original value.
How does Call by Reference work in C Programming?
In Call by Reference, the memory address of the argument is passed to the function, allowing the function to directly operate on the original data. Any changes made to the parameter inside the function affect the original value outside the function. Call by Reference is useful when you want to modify the actual data passed as an argument.
What are the advantages of Call by Value and Call by Reference in C Programming?
Call by Value: Helps in maintaining data integrity by preventing unintended modifications to the original data. It is simple and easy to understand.
Call by Reference: Allows functions to directly operate on the original data, enabling modifications to reflect outside the function. It is memory-efficient as it avoids creating copies of large data.
Conclusion
In conclusion, understanding the distinctions between Call by Value and Call by Reference in C programming is crucial for efficiently managing data and optimizing program performance. Call by Value makes a copy of the actual parameter’s value, while Call by Reference passes the actual parameter’s memory address.
When deciding between the two approaches, consider the following criteria:
1. Data Size: Call by Value is suitable for smaller data types, whereas Call by Reference is preferred for more substantial data structures to avoid excessive memory usage.
2. Resource Management: Call by Value is simpler and more secure but may incur performance overhead, while Call by Reference offers better performance but requires careful handling to prevent unintended side effects.
3. Modification: Use Call by Reference when you need to modify the actual parameter within a function, as changes made are reflected outside the function.
In practice, opt for Call by Value when dealing with basic data types and immutability is desired, and choose Call by Reference for large data structures or when modifications to the original data are necessary. By carefully considering these factors, you can leverage the strengths of each approach to enhance the efficiency and reliability of your C programs.