In graph theory, Prim’s vs Kruskal’s Algorithm are two fundamental methods for finding minimum spanning trees. Prim’s algorithm builds the tree by expanding from a starting vertex, while Kruskal’s algorithm focuses on sorting edges and adding the smallest ones. This guide explores their key differences and applications in network optimization.
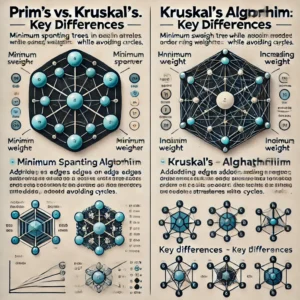
Prim’s Algorithm
Prim’s algorithm is a greedy algorithm that finds the minimum spanning tree for a connected, undirected graph. The algorithm starts with an arbitrary node and grows the tree by adding the shortest edge that connects the tree to a new node.
Example: Consider a graph with nodes A, B, C, D, and the following weighted edges: AB(2), AC(1), AD(4), BC(3), BD(2), CD(5). Starting with node A, the algorithm will add edges AC(1), AB(2), and BD(2) to form the minimum spanning tree.
Advantages:
- Efficient for dense graphs
- Easy to implement with a priority queue
Disadvantages:
- Less efficient for sparse graphs
- Requires a connected graph
Technical Characteristics:
- Time complexity: O(V^2) with adjacency matrix, O(E log V) with adjacency list
- Space complexity: O(V)
Use Cases and Applications:
- Network design
- Circuit design
Kruskal’s Algorithm
Kruskal’s algorithm is another greedy algorithm for finding the minimum spanning tree in a connected, undirected graph. The algorithm sorts all the edges in non-decreasing order of their weights and then adds edges to the tree while avoiding cycles.
Example: Using the same graph as above, Kruskal’s algorithm will select edges AC(1), AB(2), and BD(2) to form the minimum spanning tree.
Advantages:
- Efficient for sparse graphs
- Works well with disconnected graphs
Disadvantages:
- Slower for dense graphs
- Complex implementation with disjoint set data structure
Technical Characteristics:
- Time complexity: O(E log E) or O(E log V)
- Space complexity: O(V + E)
Use Cases and Applications:
- Cluster analysis
- Image segmentation
Key Differences: Prim’s vs Kruskal’s Algorithm
Prim’s Algorithm | Kruskal’s Algorithm |
---|---|
Greedy algorithm that grows a minimum spanning tree one vertex at a time | Greedy algorithm that selects edges in non-decreasing order of weight |
Uses a priority queue to select the next vertex to add to the tree | Uses a disjoint-set data structure to track connected components |
Always produces a minimum spanning tree for a connected, weighted graph | Also guarantees a minimum spanning tree for a connected, weighted graph |
Selects edges based on the minimum weight connected to the current tree | Selects edges with the smallest weight not creating a cycle |
Complexity is O(E log V) with a binary heap or Fibonacci heap | Complexity is O(E log E) or O(E log V) with efficient union-find |
Performs better on dense graphs due to efficient priority queue operations | Excels on sparse graphs due to its simplicity and edge sorting |
Not naturally parallelizable due to its sequential nature | Can be parallelized easily by processing independent edges simultaneously |
Typically requires more memory for the priority queue and vertex tracking | Generally consumes less memory, mainly for the disjoint-set data structure |
Frequently Asked Questions: Prim’s vs Kruskal’s Algorithm
What is Prim’s Algorithm and how does it work?
Prim’s Algorithm is a greedy algorithm used to find the minimum spanning tree in a weighted graph. It starts from an arbitrary vertex, grows the tree by adding the shortest edge that connects a vertex in the tree to a vertex outside the tree, and continues until all vertices are included in the tree.
How does Kruskal’s Algorithm differ from Prim’s Algorithm?
Kruskal’s Algorithm is also a greedy algorithm used to find the minimum spanning tree in a weighted graph. However, Kruskal’s Algorithm works by sorting all the edges in the graph by weight and then adding the edges one by one, avoiding cycles, until all vertices are connected.
Which algorithm is more efficient: Prim’s or Kruskal’s?
Prim’s Algorithm is generally more efficient on dense graphs with a smaller number of edges. Kruskal’s Algorithm, on the other hand, is more efficient on sparse graphs with a large number of edges. The efficiency of each algorithm also depends on the specific characteristics of the graph.
When should I choose Prim’s Algorithm over Kruskal’s Algorithm?
You should choose Prim’s Algorithm when you have a dense graph with fewer edges and when you want to start building the minimum spanning tree from a specific vertex. Prim’s Algorithm is also easier to implement when the graph is represented using an adjacency matrix.
Can Kruskal’s Algorithm be used on a weighted graph with negative edge weights?
No, Kruskal’s Algorithm cannot be directly used on a weighted graph with negative edge weights because it relies on sorting edges by weight, which may lead to incorrect results when negative weights are involved. Prim’s Algorithm can handle negative edge weights with modifications.
Conclusion
In conclusion, after delving into the intricacies of Prim’s vs Kruskal’s algorithms for finding optimal paths in graphs, several key differences have emerged. Prim’s algorithm operates by expanding a tree from a single vertex, ensuring connectivity at each step, while Kruskal’s algorithm focuses on adding edges based on weight, prioritizing minimum spanning tree construction.
Based on a thorough analysis, it is recommended to choose Prim’s algorithm for dense graphs where the number of edges is close to the maximum possible. Conversely, Kruskal’s algorithm is preferable for sparse graphs with fewer edges, as it efficiently handles disconnected components.
Decision-making criteria to consider include the graph’s density, edge weights distribution, and connectivity requirements. For dense graphs with high connectivity needs, Prim’s algorithm excels, whereas Kruskal’s algorithm is more suitable for sparse graphs with varying edge weights and disjoint components. By aligning the algorithm choice with these criteria, optimal paths can be effectively unveiled in graph theory applications.
Binary Tree vs Binary Search Tree: Key Differences Explained