Finite state machines play a critical role in digital systems, with Mealy vs Moore machines being two key types. A Mealy machine generates outputs based on the current state and inputs, while a Moore machine relies solely on the current state. This guide explores their differences and real-world applications.
Mealy Machine
A Mealy machine is a finite state machine where the outputs are dependent on both the current state and the inputs.
- Explanation: In a Mealy machine, the outputs are a function of both the current state and the inputs. The output is not only determined by the state but also by the input symbols.
- Example: Consider a vending machine that dispenses a product based on the amount entered and the selected item. The output (product dispensed) depends on both the current state (amount entered) and the input (selected item).
- Code Snippet (Pseudocode):
state currentState; function nextState(input) { currentState = transitionFunction(currentState, input); return outputFunction(currentState, input); }
Advantages:
- More efficient in terms of memory as outputs depend on inputs.
- Can produce outputs faster as they are not delayed by state transitions.
Disadvantages:
- May introduce more complex behavior due to output dependency on inputs.
- Harder to design and debug compared to Moore machines.
Moore Machine
A Moore machine is a finite state machine where the outputs are only dependent on the current state.
- Explanation: In a Moore machine, the outputs are solely determined by the current state. The output remains constant until a state transition occurs.
- Example: Traffic light control system where the lights change based on the current state of the system (green, yellow, red).
- Code Snippet (Pseudocode):
state currentState; function nextState(input) { currentState = transitionFunction(currentState, input); return outputFunction(currentState); }
Advantages:
- Easier to design and understand as outputs are solely based on states.
- Less complex due to output independence from inputs.
Disadvantages:
- May result in slower output response times due to state transitions.
- Require more memory as outputs are produced based on states only.
Technical Characteristics:
- Mealy machines have fewer states compared to Moore machines for the same functionality.
- Moore machines are more suitable for applications where output changes need to be synchronized with state transitions.
Use Cases and Applications:
- Mealy Machines: Communication protocols, vending machines, signal processing systems.
- Moore Machines: Traffic light controllers, elevator control systems, digital clocks.
Mealy vs Moore Machines: Key Differences
Mealy Machine | Moore Machine |
---|---|
Output depends on current state and input | Output depends only on current state |
More complex to design due to output dependency on inputs | Easier to design as outputs are tied to states |
Outputs change on input transition | Outputs change after state transition |
Mealy machines have a faster response time | Moore machines have more stable outputs |
Can represent systems with fine-grained control over outputs | Preferred for systems with less frequent output changes |
Transition diagram includes both inputs and outputs | Transition diagram only includes inputs |
Requires more states to handle the same functionality compared to Moore machines | May require fewer states due to output constraints |
Output values can change asynchronously with respect to input changes | Output values change synchronously with state changes |
Good for systems where actions need to be taken based on input history | Good for systems where actions are based solely on the current state |
Can be more error-prone due to potential glitches on output changes | Less susceptible to glitches in output due to state-based outputs |
Generally considered more versatile due to input-output dependency | Considered more robust in terms of avoiding output errors |
Used in applications with continuous monitoring or where rapid response is crucial | Commonly applied in systems requiring stability and consistent behavior |
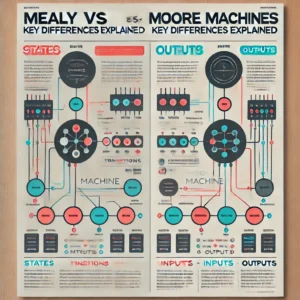
Practical Implementation
Let’s consider a simple example to illustrate the difference between Mealy and Moore machines. We’ll implement a Mealy machine to detect patterns in an input stream and a Moore machine to count the occurrences of a specific symbol.
Mealy Machine Implementation:
#include <iostream>
using namespace std;
int main() {
char input;
bool patternDetected = false;
while (true) {
cin >> input;
if (input == '1') {
patternDetected = !patternDetected;
}
if (patternDetected) {
cout << "Pattern detected!" << endl;
} else {
cout << "Waiting for pattern..." << endl;
}
}
return 0;
}
Moore Machine Implementation:
#include <iostream>
using namespace std;
int main() {
char input;
int count = 0;
while (true) {
cin >> input;
if (input == '0') {
count++;
}
cout << "Count: " << count << endl;
}
return 0;
}
Step-by-Step Implementation Guide:
- Create the necessary variables for state and input handling.
- Define the transition logic based on the current state and input for Mealy and Moore machines.
- Implement the output generation based on the current state.
- Execute the machine in a loop to continuously process input.
Best Practices and Optimization Tips:
- Optimize transition and output logic for better performance.
- Use efficient data structures to store states and inputs.
- Handle edge cases and invalid inputs gracefully.
Common Pitfalls and Solutions:
- Misunderstanding the difference between Mealy and Moore machines can lead to incorrect implementations. Ensure clarity on state transitions and output generation.
- Improper handling of input streams can cause unexpected behavior. Validate inputs and implement error handling mechanisms.
Frequently Asked Questions
What are the fundamental differences between Mealy and Moore machines?
Mealy machines output a value based on both the current state and the inputs, while Moore machines produce output solely based on the current state. This distinction is crucial as it affects the behavior and implementation of the machines.
How do Mealy and Moore machines differ in terms of output timing?
In Mealy machines, the output changes as soon as the input changes, leading to potentially faster response times. In contrast, Moore machines update their output only when transitioning between states, which can result in a delay compared to Mealy machines.
Which type of machine is more memory-efficient: Mealy or Moore?
Moore machines are generally more memory-efficient because they do not need to store information about inputs when determining the output. Mealy machines require additional memory to keep track of input conditions along with the states.
Can you explain the impact of Mealy vs. Moore machines on synchronous and asynchronous systems?
In synchronous systems, Moore machines are often preferred due to their predictable behavior and simplicity in design. On the other hand, Mealy machines are more suitable for asynchronous systems where outputs need to change immediately with input variations.
How do Mealy and Moore machines differ in terms of state transitions?
Mealy machines may have different output values even in the same state, depending on the input, leading to a more complex state transition diagram. Moore machines, however, have a single output value per state, simplifying the state transition representation.
Conclusion
In conclusion, Mealy and Moore machines are distinct in their design and functionality despite serving similar purposes in the realm of finite state machines. Mealy machines are characterized by output being dependent on both the current state and input, while Moore machines base output solely on the current state. This fundamental difference impacts their applications and efficiency in various scenarios.
When deciding between Mealy and Moore machines, it is crucial to consider the specific requirements of the system. Mealy machines are advantageous when response time is critical and the output needs to be updated swiftly based on input changes. On the other hand, Moore machines are more suitable for applications where output stability is paramount and input changes do not require immediate output adjustments.
Key decision-making criteria include the complexity of the system, performance requirements, input/output dependencies, and ease of implementation and maintenance. By carefully evaluating these factors, one can determine whether a Mealy or Moore machine is better suited for the intended application, ultimately leading to a more efficient and effective system design.