Understanding the key differences between Java Method Overloading vs. Method Overriding will help you grasp essential concepts of inheritance and polymorphism in Java.
Method Overloading
Method overloading in Java allows a class to have multiple methods with the same name but different parameters. The compiler differentiates these methods based on the number of parameters and their types. Method overloading is resolved at compile time.
Advantages:
- Improves code readability and maintainability.
- Provides flexibility in method design.
- Allows the creation of methods with different functionalities but the same name.
Disadvantages:
- May lead to confusion if overused or misused.
- Can make the code harder to understand for developers unfamiliar with the codebase.
Method Overriding
Method overriding occurs when a subclass provides a specific implementation of a method that is already provided by its superclass. It allows a subclass to provide a specialized version of a method defined in its superclass. Method overriding is resolved at runtime.
Advantages:
- Supports run-time polymorphism.
- Enables the implementation of runtime decisions based on objects’ types.
- Facilitates code reusability and flexibility in design.
Disadvantages:
- Can introduce complexity if not used carefully.
- May lead to unexpected behaviors if not fully understood.
Technical Characteristics
Method overloading is achieved within the same class, while method overriding involves a superclass-subclass relationship. Overloaded methods must have different method signatures, while overridden methods share the same signature.
Use Cases and Applications
- Method overloading is commonly used in Java libraries to provide multiple methods with similar functionalities but different numbers or types of parameters.
- Method overriding is extensively used in inheritance scenarios where subclasses need to customize the behavior of inherited methods from their superclass.
- Both concepts play a crucial role in designing flexible and extensible Java applications.
Java Method Overloading vs. Method Overriding: Key Differences
Method Overloading | Method Overriding |
---|---|
Occurs within the same class | Occurs in a parent-child class relationship |
Requires methods with the same name but different parameters | Requires methods with the same name and parameters |
Is determined at compile-time | Is determined at runtime |
Does not involve inheritance | Involves inheritance |
Provides multiple methods with the same name but different signatures | Provides a specific implementation of a method in a subclass that is already provided by its parent class |
Helps in improving code readability and reusability | Facilitates polymorphism and dynamic method invocation |
Argument list must differ in terms of number or type of parameters | Method signature must be the same including the return type |
Static polymorphism | Dynamic polymorphism |
Overloading is done in the same class | Overriding is done in two classes that have an inheritance relationship |
Compile-time polymorphism | Run-time polymorphism |
Return type may or may not be different | Return type must be the same or a covariant type |
Changes the signature of the method | Does not change the signature, but provides a new implementation |
Can be done in the same class | Must be done in sub-classes |
Does not require a parent-child relationship | Requires a parent-child relationship |
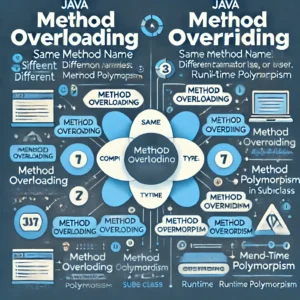
Practical Implementation
Method Overloading
Method overloading in Java allows a class to have multiple methods with the same name but different parameters.
Practical Implementation Example:
Let’s consider a class Calculator with overloaded methods for addition:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
}
Step-by-Step Implementation Guide:
- Create a Java class (e.g., Calculator).
- Define multiple methods with the same name but different parameters.
- Call the methods with different argument types to see method overloading in action.
Best Practices and Optimization Tips:
- Use method overloading to provide flexibility and convenience to users of your class.
- Avoid overloading methods with ambiguous parameter types to prevent confusion.
- Ensure that the overloaded methods have different parameter lists to distinguish them.
Common Pitfalls and Solutions:
- Pitfall: Overloading methods with the same number and type of parameters can lead to compilation errors.
- Solution: Ensure that the overloaded methods have distinct parameter lists to resolve conflicts.
Method Overriding
Method overriding in Java enables a subclass to provide a specific implementation of a method that is already provided by its superclass.
Practical Implementation Example:
Consider a class Shape with a method calculateArea() that is overridden in subclasses:
public class Shape {
public double calculateArea() {
return 0;
}
}
public class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double calculateArea() {
return Math.PI radius radius;
}
}
Step-by-Step Implementation Guide:
- Create a superclass with a method to be overridden.
- Create a subclass that extends the superclass.
- Override the method in the subclass using the @Override annotation.
Best Practices and Optimization Tips:
- Use method overriding to provide specific behavior for a method in subclasses.
- Ensure that the overridden method has the same signature (name and parameters) as the superclass method.
- Consider using the super keyword to call the superclass method within the overridden method if needed.
Common Pitfalls and Solutions:
- Pitfall: Changing the method signature in the subclass when overriding can lead to compilation errors.
- Solution: Make sure the overridden method has the same signature as the superclass method to avoid conflicts.
Frequently Asked Questions
What is method overloading in Java?
Method overloading in Java allows a class to have multiple methods with the same name but different parameters. The compiler determines which method to execute based on the number and type of arguments passed during the method call.
What is method overriding in Java?
Method overriding in Java occurs when a subclass provides a specific implementation for a method that is already defined in its superclass. The method in the subclass must have the same signature (name, return type, and parameters) as the method in the superclass.
How does Java determine which method to invoke in method overloading?
In method overloading, Java determines which method to invoke during compile time based on the number and type of arguments passed. The method with the most specific parameter list that matches the arguments provided will be executed.
Can method overriding be done in the same class in Java?
No, method overriding in Java can only occur between a superclass and its subclass. The overridden method must have the same name, return type, and parameters in both classes to achieve polymorphic behavior.
What is the main difference between method overloading and method overriding in Java?
Method overloading allows a class to have multiple methods with the same name but different parameters, while method overriding occurs when a subclass provides a specific implementation for a method already defined in its superclass with the same signature.
Conclusion
In conclusion, grasping the key differences between Java Method Overloading vs. Method Overriding is essential for mastering object-oriented programming concepts. Method overloading involves defining multiple methods with the same name but different parameters within the same class, while method overriding is the process of creating a new implementation of a method in a subclass that has the same signature as a method in its superclass.
Key differences between method overloading and method overriding include:
1. Method overloading occurs within the same class, while method overriding involves a subclass and its superclass.
2. Method overloading is resolved at compile time based on the method signature, whereas method overriding is resolved at runtime based on the actual object type.
In practice, when deciding whether to use method overloading or method overriding, consider the following criteria:
- Use method overloading when you want to provide multiple ways to invoke a method with different parameters.
- Use method overriding when you want to redefine the behavior of a method in a subclass to suit its specific requirements or when implementing polymorphism.
By carefully evaluating your design requirements and considering the specific use case, you can determine whether method overloading or method overriding is more appropriate for your Java programming needs. Mastering these concepts will enhance your programming skills and enable you to create more efficient and maintainable code in Java.