Functional Components
Functional components are simple functions that accept props as arguments and return React elements. They are stateless, meaning they do not manage their own state.
- Easy to read and understand
- Lightweight and simple to implement
- Performance optimizations by React through the use of hooks
Example:
import React from 'react'; const FunctionalComponent = (props) => { return (
); }; export default FunctionalComponent;
Class Components
Class components are ES6 classes that extend from React.Component
. They have a built-in state object and lifecycle methods.
- Can manage state and have lifecycle methods
- More feature-rich and versatile
- Historically used before the introduction of hooks
Example:
import React, { Component } from 'react';
class ClassComponent extends Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
render() {
return
;
}
}
export default ClassComponent;
Advantages and Disadvantages
Functional Components
Advantages:
- Simple and easier to understand
- Performance optimizations with React hooks
Disadvantages:
- Can’t use state or lifecycle methods directly (before hooks)
Class Components
Advantages:
- Can manage state and use lifecycle methods
- More feature-rich and versatile
Disadvantages:
- More boilerplate code
- Complexity increases with larger applications
Technical Characteristics
Functional Components:
- Stateless
- Use hooks for managing state and side effects
Class Components:
- Have a built-in state object
- Use lifecycle methods for managing component behavior
Use Cases and Applications
Functional components are suitable for simple UI components or when managing state is not required. Class components are better suited for complex components that require state management and lifecycle methods.
Key Differences between Functional and Class
Functional Components | Class Components |
---|---|
Based on JavaScript functions | Defined using ES6 classes |
No ‘this’ keyword | Uses ‘this’ keyword to access props and state |
Stateless, no internal state management | Can have internal state using this.state |
Don’t support lifecycle methods | Supports lifecycle methods like componentDidMount |
Functional and easier to read for simple components | More verbose, suitable for complex components |
Can use hooks like useEffect and useState since React 16.8 | Cannot directly use hooks, needs to be converted to functional component |
Performance optimizations handled by React | May require manual optimizations for performance improvement |
Functional components are often stateless presentational components | Class components are used for more feature-rich components |
Easy to test as they are pure functions | Testing may be more complex due to state and lifecycle |
Can use arrow functions for event handlers directly | Need to bind event handlers in the constructor for ‘this’ context |
Encourages functional programming concepts | Enforces OOP principles |
Functional components are more lightweight | Class components are heavier due to the additional features |
Readability is high due to less boilerplate code | Can be verbose and have more boilerplate |
Component logic is encapsulated in functions | Component logic is spread across methods |
Can’t use lifecycle methods directly | Lifecycle methods allow more control over component behavior |
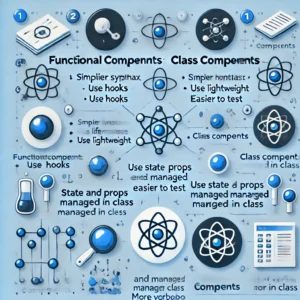
Practical Implementation
Detailed practical implementation examples
Functional components are simple JavaScript functions that accept props as arguments and return React elements. On the other hand, class components are ES6 classes that extend React.Component and have a render method to return React elements.
Working code snippets
Functional Component Example:
function FunctionalComponent(props) {
return (
{props.title}
{props.description}
);
}
Class Component Example:
class ClassComponent extends React.Component {
render() {
return (
{this.props.title}
{this.props.description}
);
}
}
Step-by-step implementation guide
1. Define a functional component by creating a JavaScript function that returns JSX.
2. Pass props as arguments to the function to access data.
3. Define a class component by creating a class that extends React.Component.
4. Implement a render method inside the class component to return JSX.
5. Access props using this.props in class components.
Best practices and optimization tips
- Use functional components for simpler UI components.
- Use class components for complex components that require state or lifecycle methods.
- Prefer functional components for better performance as they are lighter.
- Utilize React Hooks in functional components for managing state and side effects.
Common pitfalls and solutions
Pitfall: Forgetting to bind this in class component methods.
Solution: Use arrow functions to automatically bind this or explicitly bind this in the constructor.
Pitfall: Using lifecycle methods in functional components.
Solution: Use useEffect hook for managing side effects in functional components.
Pitfall: Mixing functional and class components in the same project.
Solution: Choose a consistent approach for component types across the project to maintain code consistency.
By understanding the differences between functional and class components and their appropriate use cases, you can effectively implement React components in your projects.
Frequently Asked Questions
What are functional components in React?
Functional components in React are JavaScript functions that take in props as arguments and return React elements to describe what should appear on the screen. They are simple and lightweight, making them easy to understand and write.
What are class components in React?
Class components in React are ES6 classes that extend from React.Component. They have a render() method that returns React elements to render on the screen. Class components have more features like state and lifecycle methods compared to functional components.
What is the main difference between functional and class components?
The main difference is how state and lifecycle methods are handled. Functional components are stateless and do not have their own state or lifecycle methods. Class components, on the other hand, can hold state and define lifecycle methods like componentDidMount and componentDidUpdate.
When should I use functional components?
Functional components should be used when you have simple presentation components that do not require state or lifecycle methods. They are easy to test and understand, promoting a more functional programming style in your React applications.
When should I use class components?
Class components should be used when you need to manage state or use lifecycle methods in your components. If you are working on a complex UI component that needs to handle state changes or fetch data from an API, class components provide the necessary features and flexibility.
Conclusion
In conclusion, the comparison between functional and class components sheds light on their key differences. Functional components are simpler, more concise, and utilize hooks for state management, while class components are more traditional, have lifecycle methods, and use this keyword for state handling.
Based on this analysis, it is recommended to opt for functional components for new projects due to their modern approach, better performance, and the industry trend favoring functional programming. However, for legacy codebases or situations where lifecycle methods are essential, using class components might be necessary.
Decision-making criteria should include the project requirements, team expertise, scalability needs, and future maintenance considerations. By weighing these factors, developers can make informed choices between functional and class components to ensure optimal performance and code sustainability.