Map
The map container in C++ is an ordered associative container that stores elements formed by a combination of a key-value pair. It is implemented as a balanced binary search tree, usually a red-black tree, which ensures logarithmic time complexity for most of its operations.
Example:
#include
Advantages:
- Elements are ordered based on the key.
- Supports operations like finding, insertion, and deletion with logarithmic complexity.
Disadvantages:
- Slower insertion and deletion compared to unordered_map.
Unordered_map
The unordered_map container is an unordered associative container that stores elements formed by a combination of a key-value pair. It uses a hash table to store the elements, which provides constant time complexity for average-case operations.
Example:
#include
int main() {
std::unordered_map<int, std::string> myUnorderedMap;
myUnorderedMap[1] = "apple";
myUnorderedMap[2] = "banana";
// ...
}
Advantages:
- Constant-time complexity for most operations on average.
- Efficient for large datasets.
Disadvantages:
- Does not maintain the order of elements based on the key.
Use Cases and Applications
Map: Use map when you need to maintain the order of elements based on the key and when logarithmic complexity for operations is acceptable.
Unordered_map: Use unordered_map when you require constant-time complexity for most operations, especially for large datasets, and when the order of elements is not important.
Key Differences Between C++ map and unordered_map
C++ map | C++ unordered_map |
---|---|
Ordered container | Unordered container |
Implemented using Red-Black Tree | Implemented using Hash Table |
Slower lookup time O(log n) | Faster lookup time O(1) on average |
Preserves the order of elements | Does not preserve the order of elements |
Insertion time complexity is O(log n) | Insertion time complexity is O(1) on average |
Iterating over elements follows the order | Iterating over elements may not follow any specific order |
Slower for large datasets due to balancing operations | More efficient for large datasets due to constant time complexity |
Useful when iteration order is important | Useful when fast lookups are critical |
Higher memory consumption due to node structure | Lower memory consumption due to hashing |
Lower worst-case time complexity for lookups (O(log n)) | No worst-case time complexity for lookups (O(1) on average) |
May have better cache locality for smaller datasets | May have poorer cache locality due to hash table resizing |
Slower for search, insertion, and deletion | Faster for search, insertion, and deletion |
Provides stable iterators even after insertions | Iterators may become invalid after insertions |
Requires less work to maintain order in elements | Offers more predictable performance for most operations |
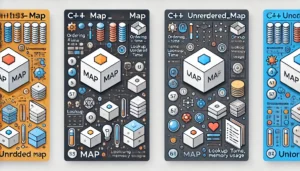
Practical Implementation
C++ provides two widely-used associative containers for storing key-value pairs: std::map and
std::unordered_map. Each has its own strengths and weaknesses, making it important to choose the
right one based on your specific requirements.
Map Implementation Example:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap;
myMap[1] = "One";
myMap[2] = "Two";
myMap[3] = "Three";
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
Unordered_map Implementation Example:
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, std::string> myUnorderedMap;
myUnorderedMap[1] = "One";
myUnorderedMap[2] = "Two";
myUnorderedMap[3] = "Three";
for (const auto& pair : myUnorderedMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
Step-by-step Implementation Guide
- Include the relevant header file:
#include <map>
for std::map and
#include <unordered_map>
for std::unordered_map.
- Create an instance of the desired container with the appropriate key and value types.
- Insert key-value pairs using the
operator[]
orinsert
method. - Iterate over the container to access and process the stored key-value pairs.
Best Practices and Optimization Tips
- Use std::map when you need a sorted associative container with logarithmic lookup complexity.
- Prefer std::unordered_map for faster average constant-time lookups but with no specific order.
- Consider the memory overhead of std::unordered_map due to hash tables.
- Choose the appropriate container based on your specific usage patterns and requirements.
Common Pitfalls and Solutions
- Pitfall: Forgetting to include the necessary header file before using std::map
or std::unordered_map. - Solution: Always include
#include <map>
or#include <unordered_map>
at the beginning of your program. - Pitfall: Incorrectly using the container methods, leading to unexpected behavior or errors.
- Solution: Refer to the C++ standard library documentation for proper usage and syntax of container methods.
Frequently Asked Questions
What is the difference between C++’s map and unordered_map?
C++’s map is a container that stores key-value pairs in a sorted order based on the keys, while unordered_map stores key-value pairs in an unordered fashion using a hash function.
When should I use a map instead of an unordered_map?
Use map when you need the keys to be sorted, want to iterate over elements in a sorted order, or require operations like finding elements by key or range-based queries.
When is an unordered_map more suitable than a map?
Choose unordered_map when the order of keys is not important, you need faster average access times, or when you don’t require the keys to be stored in a specific sorted order.
Does the choice between map and unordered_map affect performance?
Yes, the choice between map and unordered_map can significantly impact performance. unordered_map generally provides faster access times for lookups, insertions, and deletions due to its hash-based implementation.
Can I use custom objects as keys in both map and unordered_map?
Yes, you can use custom objects as keys in both map and unordered_map by defining custom comparison functions or hash functions, depending on the container you choose.
Conclusion
In conclusion, when comparing C++’s map and unordered_map, several key differences stand out. The map is a red-black tree-based associative container that maintains a sorted order of elements by key, providing logarithmic time complexity for most operations. On the other hand, the unordered_map is a hash table-based container that offers constant time complexity for average case operations but does not maintain any specific order of elements.
For users seeking ordered traversal, key uniqueness, and a standard library implementation, the map is the way to go. Its logarithmic time complexity guarantees efficient operations for most scenarios, especially when the data set is small or the ordering of elements is crucial.
Conversely, if constant time complexity and faster average performance for insertion, deletion, and search operations are priorities, the unordered_map is the better choice. Its hash table implementation excels in scenarios where order is not important, and the focus is on fast access to key-value pairs in large data sets.
When deciding between map and unordered_map, consider the following criteria:
1. Data requirements: Determine if ordered traversal or constant time complexity is more critical for your specific use case.
2. Performance needs: Assess whether the logarithmic time complexity of the map or the constant time complexity of the unordered_map better suits your application’s performance demands.
3. Memory considerations: Consider the memory overhead of maintaining order in the map versus the potential space efficiency of the unordered_map.
4. Compatibility: Evaluate the compatibility of the container type with your existing codebase and library dependencies.
Ultimately, the choice between map and unordered_map hinges on your specific requirements and trade-offs between performance, memory usage, and functionality. By carefully evaluating these factors, you can select the right container that best aligns with your project’s objectives and constraints.