Deterministic Finite Automaton (DFA)
A Deterministic Finite Automaton is a finite state machine where for each input symbol, there is exactly one transition defined. It processes inputs sequentially and reaches a unique state for a given input.
Example of a simple DFA:
State Input: 0 Input: 1 -------------------------------- q0 q1 q0 q1 q2 q0 q2 q1 q0
Advantages:
- Deterministic nature leads to easier implementation and analysis.
- Only one possible transition for each input symbol, making it predictable.
- Efficient in terms of memory and processing as it has a fixed number of states.
Disadvantages:
- Cannot handle ambiguity in transitions.
- May require more states to represent certain languages.
Non-Deterministic Finite Automaton (NFA)
An Non-Deterministic Finite Automaton is a finite state machine where for a given input symbol, there can be multiple transitions or no transitions defined. It can be in several states simultaneously for a given input.
Example of a simple NFA:
State Input: 0 Input: 1 -------------------------------- q0 q0, q1 q0 q1 ∅ q2 q2 q2 q2
Advantages:
- Allows more flexibility due to non-deterministic transitions.
- Can represent certain languages with fewer states compared to DFA.
Disadvantages:
- Complexity in implementation and analysis due to non-deterministic behavior.
- Requires additional processing to handle multiple transitions for a given input.
- Technical Characteristics
Deterministic Finite Automaton and Non-Deterministic Finite Automaton differ in terms of their transition functions and behavior when processing inputs. DFA has a deterministic transition function, while NFA can have non-deterministic transitions.
Use Cases and Applications
Both DFA and NFA find applications in various areas such as lexical analysis, pattern matching, parsing, and network protocols.
DFA is commonly used in compilers for tokenization and syntax analysis due to its deterministic nature, while NFA is often employed in regular expression matching algorithms for its flexibility in representing patterns.
DFA vs NFA Key Differences
DFA (Deterministic Finite Automaton) | NFA (Nondeterministic Finite Automaton) |
---|---|
Transition function is uniquely determined for each state and input symbol combination. | Transition function may lead to multiple possible states for the same input symbol and current state. |
Conversion from NFA to DFA is generally more complex. | Conversion from DFA to NFA is relatively simpler. |
Requires more memory as it needs to store information for each state transition. | Typically requires less memory as it can utilize epsilon transitions and do not need to track all possible transitions. |
DFA is more suitable for implementing lexical analyzers. | NFA is more commonly used for pattern matching algorithms. |
Every input symbol must have a defined transition for all states in DFA. | Input symbol may have undefined transitions for some states in NFA. |
Deterministic nature leads to deterministic behavior for the same input. | Nondeterministic nature allows for different paths for the same input. |
Transition from one state to another is always uniquely defined. | Transition may lead to multiple states simultaneously in NFA. |
Complexity in handling languages with multiple transitions or states. | More flexibility in representing languages due to nondeterministic behavior. |
Usually easier to understand and analyze due to deterministic behavior. | Can be harder to predict behavior due to multiple possible transitions. |
Guarantees to accept/reject input strings unequivocally. | Acceptance of input strings can be based on multiple possible outcomes. |
Transition between states is based on a single input symbol. | Transitions can be based on multiple input symbols or epsilon transitions. |
Regular operations such as intersection, union, and complement are more straightforward to implement. | Regular operations may require additional steps due to the nondeterministic nature. |
Deterministic behavior simplifies debugging and testing. | Nondeterministic behavior can make debugging more challenging. |
The number of states in DFA may be larger due to determinism. | NFA can sometimes have fewer states due to its nondeterministic nature. |
DFA guarantees unique outcomes for every input string. | NFA can have multiple paths leading to acceptance for the same input string. |
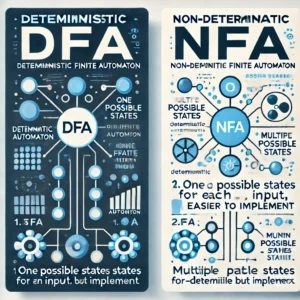
Practical Implementation
DFA vs. NFA: Practical Implementation Examples
Let’s consider a scenario where we need to implement a simple string pattern matcher using a Deterministic Finite Automaton (DFA) and a Non-deterministic Finite Automaton (NFA).
DFA Implementation
class DFA:
def __init__(self, transitions, start_state, accept_states):
self.transitions = transitions
self.current_state = start_state
self.accept_states = accept_states
def process_input(self, input_string):
for char in input_string:
self.current_state = self.transitions.get((self.current_state, char))
if self.current_state is None:
return False
return self.current_state in self.accept_states
DFA transitions for matching 'abc'
transitions_dfa = {
(0, 'a'): 1,
(1, 'b'): 2,
(2, 'c'): 3
}
dfa = DFA(transitions_dfa, 0, {3})
print(dfa.process_input('abc')) Output: True
NFA Implementation
class NFA:
def __init__(self, transitions, start_state, accept_states):
self.transitions = transitions
self.current_states = {start_state}
self.accept_states = accept_states
def process_input(self, input_string):
for char in input_string:
next_states = set()
for state in self.current_states:
next_states.update(self.transitions.get((state, char), set()))
self.current_states = next_states
return bool(self.current_states.intersection(self.accept_states))
NFA transitions for matching 'abc'
transitions_nfa = {
(0, 'a'): {1},
(1, 'b'): {2},
(2, 'c'): {3}
}
nfa = NFA(transitions_nfa, 0, {3})
print(nfa.process_input('abc')) Output: True
Step-by-Step Implementation Guide
- Create the transition functions for DFA and NFA.
- Define the initial states and accept states.
- Implement the process_input method to simulate the automaton.
- Test the implementations with sample input strings.
Best Practices and Optimization Tips
- Use efficient data structures like dictionaries to store transitions for faster lookups.
- Optimize transition functions to reduce redundant states and transitions.
- Consider minimizing the automata to reduce the number of states and transitions.
Common Pitfalls and Solutions
- Common Pitfall: Incorrect transition definitions leading to incorrect matching.
- Solution: Double-check transition definitions and test with various input strings to ensure correct behavior.
- Common Pitfall: Not handling epsilon transitions correctly in NFAs.
- Solution: Implement epsilon closure to handle epsilon transitions effectively.
Frequently Asked Questions
What is the main difference between DFA and NFA?
The key difference lies in their design complexity. A DFA has a deterministic transition for each input symbol in each state, while an NFA allows multiple transitions from a state for the same input symbol.
Why are NFAs often considered more flexible than DFAs?
NFAs can have ε-transitions (epsilon-transitions) that allow them to transition to the next state without consuming any input. This feature makes NFAs more flexible in representing certain types of languages or patterns.
Can a DFA recognize all languages that an NFA can recognize?
Yes, a DFA can recognize all languages that an NFA can recognize. This is due to the fact that any NFA can be converted to an equivalent DFA using methods like the subset construction.
Which one is generally easier to design and analyze: DFA or NFA?
DFAs are typically easier to design and analyze than NFAs because of their deterministic nature. DFAs have clear transitions for each input symbol in each state, which simplifies the understanding of their behavior.
How does the acceptance criterion differ between DFA and NFA?
In a DFA, acceptance is based on reaching a designated accepting state after processing the entire input string. In an NFA, acceptance can be based on reaching any accepting state through one of the possible computation paths.
Conclusion
In conclusion, the key differences between Deterministic Finite Automata (DFA) and Non-deterministic Finite Automata (NFA) lie in their design complexity and computational capabilities. DFAs are simpler to understand and implement, while NFAs offer greater flexibility but are more complex to analyze.
When deciding between DFA and NFA, it is important to consider the specific requirements of the problem at hand. If a clear and deterministic solution is sufficient, DFA may be the better choice due to its simplicity and efficiency. However, if the problem involves non-deterministic elements or requires more complex logic, NFA could provide a more suitable solution.
The decision-making criteria should include the complexity of the problem, the need for flexibility in handling non-deterministic inputs, and the computational resources available. By carefully evaluating these factors, one can make an informed choice between DFA and NFA to effectively address the unique requirements of the given problem.