Structure in C++
A structure in C++ is a user-defined data type that allows you to group different variables of various data types under a single name. It is primarily used for representing records.
Example:
struct Person { string name; int age; };
Advantages:
- Simple and easy to use
- Memory-efficient
- Can be used to represent a record with different data types
Disadvantages:
- Lacks data encapsulation and does not support member functions
- No access control modifiers such as public and private
Class in C++
A class in C++ is also a user-defined data type that allows you to group data members and member functions under a single name. It provides the concept of data abstraction and encapsulation.
Example:
class Circle { private: double radius; public: void setRadius(double r) { radius = r; } double getArea() { return 3.14 radius radius; } };
Advantages:
- Supports data encapsulation and data hiding
- Allows inheritance and polymorphism
- Can have member functions for better organization of code
Disadvantages:
- Can be more complex and harder to understand compared to structures
- May introduce overhead due to the use of member functions
Use Cases and Applications
Structures are commonly used when you need a simple way to group related data together, such as representing a point in 2D space or storing information about a student. Classes, on the other hand, are used in more complex scenarios where you need to model real-world entities with behaviors and attributes.
Key Differences between Structure vs Class in C++
Structure | Class |
---|---|
Does not have member functions | Can have member functions |
Cannot have access specifiers | Can have public, private, and protected access specifiers |
Used for organizing data elements | Used for organizing data and functions as a single unit |
Cannot be inherited | Can be inherited |
Cannot be initialized at the point of declaration | Can be initialized at the point of declaration |
Cannot have constructors or destructors | Can have constructors and destructors |
Size is not fixed | Size is fixed |
Cannot contain member functions | Can contain member functions |
Can be used for simple data structures | Can be used for more complex data structures with behavior |
Does not support polymorphism | Supports polymorphism |
Does not support method overloading | Supports method overloading |
Cannot define constants | Can define constants using ‘const’ keyword |
Does not support operator overloading | Supports operator overloading |
Cannot hide data members | Can hide data members using access specifiers |
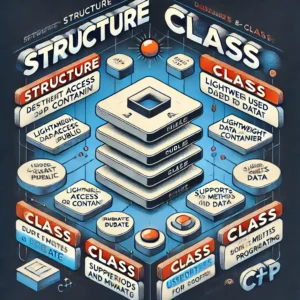
Practical Implementation
In C++, structs and classes define custom data types, but they differ in default access specifiers and member functions. Explore practical examples to understand how structures and classes in C++ contrast with each other.
Structure Example
A structure is a user-defined data type that can hold multiple data members of different data types. By default, all members of a structure are public.
struct Person {
std::string name;
int age;
void displayInfo() {
std::cout << "Name: " << name << ", Age: " << age << std::endl;
}
};
int main() {
Person person1;
person1.name = "Alice";
person1.age = 30;
person1.displayInfo();
return 0;
}
Class Example
A class is similar to a structure but has additional features like data hiding and member functions. By default, all members of a class are private.
class Car {
private:
std::string brand;
int year;
public:
Car(std::string b, int y) : brand(b), year(y) {}
void displayInfo() {
std::cout << "Brand: " << brand << ", Year: " << year << std::endl;
}
};
int main() {
Car car1("Toyota", 2021);
car1.displayInfo();
return 0;
}
Step-by-Step Implementation Guide
- Define a structure using the
struct
keyword for simple data containers. - Define a class using the
class
keyword for more complex data types with member functions. - Access members of a structure directly since they are public by default.
- Use member functions to access and manipulate data members of a class.
Best Practices and Optimization Tips
- Use structures for simple data containers without member functions.
- Use classes for more complex data types that require data encapsulation and abstraction.
- Avoid exposing class members directly; prefer encapsulation through private data members and public member functions.
Common Pitfalls and Solutions
One common pitfall is forgetting to specify access specifiers in classes, leading to unexpected member visibility. Always explicitly define the access specifiers (public
, private
, protected
) in classes to maintain code clarity and prevent unintended access.
Frequently Asked Questions
What is the difference between structure and class in C++?
In C++, a structure is a user-defined data type that can contain variables and functions known as members, whereas a class is a more advanced version of a structure that allows encapsulation, inheritance, and polymorphism.
When should I use a structure over a class in C++?
Use a structure in C++ for small data structures with a few members that don’t need advanced features like inheritance or access control. Opt for classes when you need greater control over data access and want to utilize features such as data hiding and function overloading.
Can a structure have member functions in C++?
Yes, in C++, a structure can have member functions just like a class. This allows you to encapsulate data and behavior within a structure, making it more versatile and powerful than a simple collection of data members.
What are the key advantages of using classes over structures in C++?
One key advantage of using classes over structures in C++ is the ability to implement object-oriented programming concepts like inheritance and polymorphism. Classes also provide better data security through access specifiers like private and protected, allowing for better control over data access and manipulation.
How does memory allocation differ between structures and classes in C++?
In C++, memory allocated for a structure is contiguous for all its members, while memory allocated for a class may not be contiguous due to the presence of virtual functions and inheritance. This can impact the size and memory layout of objects, affecting performance and memory utilization.
Conclusion
In conclusion, understanding the contrast between structure vs class in C++ is essential for building efficient and organized programs. While structures focus on simple data organization, classes offer advanced features like inheritance and polymorphism, enabling more robust programming designs.
When deciding between using structures or classes in C++, consider the complexity and functionality requirements of your program. If you need a simple data structure with minimal functionality, a structure may suffice. However, if you anticipate the need for more complex behavior and relationships between data elements, opting for a class would be more appropriate.
Decision-making criteria should include the level of abstraction needed, the reusability of code, and the scalability of the program. Structures are often preferred for small, straightforward programs, while classes are better suited for larger projects that require a higher degree of modularity and extensibility.
By carefully evaluating the specific needs of your program and weighing the benefits of structures versus classes, you can make informed decisions that enhance the overall design and efficiency of your C++ code.