However, they serve different purposes and exhibit distinct characteristics.
File Systems
A file system is a method for storing, organizing, and accessing files on a storage medium such as a hard drive. It provides a hierarchical structure for arranging files and directories.
Example:
In a file system, you can create directories like “Documents” and store files such as “report.docx” within them.
Advantages of File Systems:
- Simple and straightforward to use.
- Direct access to files for reading and writing.
- Quick and efficient for basic file management tasks.
Disadvantages of File Systems:
- Lack of data integrity and consistency controls.
- Difficult to maintain in large-scale applications.
- No support for complex data relationships.
Technical Characteristics:
- Primarily used for storing unstructured data.
- Relies on the underlying operating system for managing files.
- Accessed through file paths and handles.
Use Cases and Applications:
- Storing user documents on a personal computer.
- Managing configuration files for applications.
- Storing media files like images and videos.
Databases
A database is a structured collection of data that is organized for efficient retrieval, storage, and manipulation. It employs a relational model to establish relationships between data entities.
Example:
In a database, you can have tables like “Customers” and “Orders” linked by a common key such as customer ID.
Advantages of Databases:
- Enforce data integrity through constraints and transactions.
- Enable complex queries for data analysis and reporting.
- Support scalability and concurrent access by multiple users.
Disadvantages of Databases:
- Require specialized knowledge for setup and maintenance.
- Can be resource-intensive in terms of storage and processing.
- Complexity in managing data relationships and normalization.
Technical Characteristics:
- Structured storage using tables and rows.
- Supports querying languages like SQL for data manipulation.
- ACID properties ensure data consistency and reliability.
Use Cases and Applications:
- Managing customer data in an e-commerce system.
- Storing financial transactions in a banking application.
- Analyzing and reporting on sales data in a business intelligence tool.
Key Differences: File vs Database System
File Systems | Databases |
---|---|
Primarily manage individual files and directories | Store and manage structured data in tables |
Optimized for read/write speeds and efficient access to files | Optimized for querying and processing large volumes of data |
Do not support complex querying capabilities | Support SQL queries for data retrieval and manipulation |
Accessed at a lower level with basic operations like read, write, delete | Accessed at a higher level using SQL queries for data operations |
Less structured data organization with files stored in a hierarchical structure | Structured data organization with relationships between tables |
File systems are suitable for storing unstructured data like documents, images | Databases are better for storing structured data like customer records, transactions |
Usually faster for simple file operations | May have slower performance for simple file read/write operations due to additional processing |
Minimal security features like file permissions | Robust security features including user authentication and role-based access control |
Backup and recovery processes are simpler | Backup and recovery processes are more complex and involve transaction logs |
Scalability is limited by hardware and file system design | Scalability is more flexible with options for horizontal and vertical scaling |
Less overhead in terms of memory and processing resources | Higher overhead due to additional functionalities like indexing and query optimization |
Designed for local file storage and small-scale data management | Designed for centralized data storage and handling large datasets |
Difficulty in maintaining consistency in data across multiple systems | Supports transactions to ensure data consistency and atomicity |
Primarily used for content storage and retrieval purposes | Primarily used for efficient data management and retrieval |
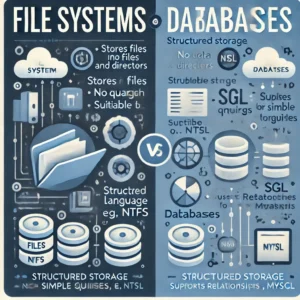
Practical Implementation
Examples
When it comes to practical implementation, let’s consider a scenario where we need to store user information such as name, email, and age. We will compare how this data can be managed using both a file system approach and a database approach.
File System Implementation
const fs = require('fs');
// Write user data to a file
const userData = { name: 'John Doe', email: 'johndoe@example.com', age: 30 };
fs.writeFileSync('userData.txt', JSON.stringify(userData));
// Read user data from the file
const fileData = fs.readFileSync('userData.txt', 'utf8');
const parsedData = JSON.parse(fileData);
console.log(parsedData);
Database Implementation
const mysql = require('mysql');
// Setup database connection
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
password: 'password',
database: 'user_data'
});
// Insert user data into the database
const userData = { name: 'Jane Smith', email: 'janesmith@example.com', age: 25 };
connection.connect();
connection.query('INSERT INTO users SET ?', userData, function (error, results, fields) {
if (error) throw error;
console.log('User data inserted successfully');
});
connection.end();
Step-by-Step Implementation Guide
- For a file system approach, use Node.js fs module to write and read data from files.
- For a database approach, set up a database connection and use query methods to interact with the database.
Best Practices and Optimization Tips
- File System: Use asynchronous file operations to prevent blocking the event loop in Node.js.
- Database: Utilize connection pooling to efficiently manage database connections and improve performance.
Common Pitfalls and Solutions
- File System Pitfall: Limited scalability due to file system constraints. Solution: Consider using a database for better scalability.
- Database Pitfall: Data integrity issues if proper constraints are not enforced. Solution: Implement database constraints and validations to maintain data integrity.
Frequently Asked Questions
What is the main difference between file systems and databases?
In a file system, data is stored in files and directories, organized in a hierarchical structure. A database, on the other hand, stores data in a structured format using tables with rows and columns. Databases provide better data management capabilities compared to file systems.
How do file systems and databases differ in terms of data retrieval?
File systems require manual searching and parsing of files to retrieve data, which can be time-consuming. Databases offer efficient query mechanisms that allow users to retrieve specific data using SQL queries, making data retrieval faster and more accurate.
Which one is better for handling structured data: file systems or databases?
Databases are more suitable for handling structured data due to their ability to enforce data integrity through constraints, relationships, and normalization. File systems lack these features and are more suited for storing unstructured data like documents and media files.
What are the scalability differences between file systems and databases?
Databases are designed for scalability, allowing for efficient management of large volumes of data with features like indexing, partitioning, and replication. File systems may struggle to handle scalability issues as the data grows, leading to performance bottlenecks and data management challenges.
How do file systems and databases differ in terms of data integrity and security?
Databases offer robust data integrity features such as ACID properties (Atomicity, Consistency, Isolation, Durability) and role-based access control for security. File systems have limited data integrity checks and security measures, making databases a more secure option for critical data storage and management.
Conclusion
In conclusion, the comparison between file systems and databases reveals distinct differences that set them apart in terms of structure, functionality, and performance. File systems are ideal for simple data storage and retrieval, whereas databases offer advanced features like data integrity, security, and scalability.
Based on these disparities, organizations must carefully consider their specific needs and requirements before deciding between file systems and databases. For small-scale projects with minimal data management needs, a file system may suffice due to its simplicity and ease of use. However, for larger and more complex applications that demand robust data handling capabilities, opting for a database is recommended.
The decision-making criteria should involve factors such as data volume, access patterns, security requirements, performance expectations, and future scalability. By evaluating these aspects thoroughly, businesses can make an informed choice between file systems and databases that aligns with their objectives and ensures efficient data management practices.