In networking, connection-oriented vs connection-less services determine how data is transmitted. Connection-oriented communication, like TCP, establishes a dedicated path for reliable data transfer, while connection-less communication, like UDP, sends data without a fixed connection. This guide explores their differences, advantages, and applications.
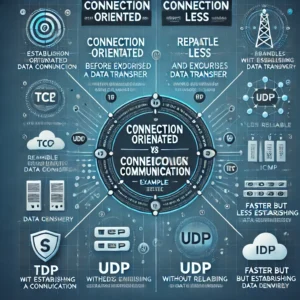
Connection-Oriented:
Connection-oriented communication requires establishing a connection between the sender and the receiver before transferring data. It ensures that data packets are delivered in order and without errors. This method relies on data acknowledgment and retransmission to guarantee successful transmission.
Advantages of Connection-Oriented:
- Reliable data delivery
- Guaranteed packet sequence
- Error detection and correction
Disadvantages of Connection-Oriented:
- Overhead due to connection setup
- Less suitable for real-time applications
Technical Characteristics:
- Uses Virtual Circuit Switching
- Transmission Control Protocol (TCP) is a common example
Use Cases and Applications:
Connection-oriented services are ideal for applications where data integrity and reliability are crucial, such as file transfers, email communication, and web browsing.
Connection-Less:
Connection-less communication does not require an established connection before transmitting data. Each packet is treated independently and sent to its destination without an acknowledgment process. This method is faster but does not guarantee the order of packet delivery.
Advantages of Connection-Less:
- Lower overhead due to no connection setup
- Suitable for real-time applications
Disadvantages of Connection-Less:
- No guaranteed packet sequence
- Possible packet loss without retransmission
Technical Characteristics:
- Uses Datagram Packet Switching
- User Datagram Protocol (UDP) is a common example
Use Cases and Applications:
Applications that prioritize speed over reliability, such as video streaming, online gaming, and Voice over IP (VoIP) communication, prefer connectionless services.
Key Differences: Connection-Oriented vs Connection-Less
Connection-Oriented | Connection-Less |
---|---|
Establishes a connection before data transfer | No connection setup is needed before data transfer |
Reliable data transfer with acknowledgment and error control | Less reliable data transfer without acknowledgment |
Order of data packets is maintained | No guarantee on the order of data packets |
Connection state is maintained throughout the communication | No connection state is stored |
Slower due to connection setup and maintenance overhead | Faster due to no connection overhead |
Commonly used in TCP (Transmission Control Protocol) | Commonly used in UDP (User Datagram Protocol) |
More suitable for applications requiring guaranteed delivery of data | More suitable for applications where speed is prioritized over reliability |
Higher protocol overhead due to reliability mechanisms | Lower protocol overhead for faster transmission |
Good for streaming media, web browsing, and email | Good for online gaming, video conferencing, and VoIP |
Complex error recovery mechanisms are in place | Simple error detection but no automatic recovery mechanisms |
Requires more resources for maintaining connections | Requires fewer resources due to stateless nature |
Packet delivery is guaranteed | No guarantee of packet delivery |
Offers flow control mechanisms | Less control over flow of data |
Supports congestion control algorithms | No inherent congestion control mechanisms |
Practical Implementation
Connection-Oriented: A practical example of a connection-oriented service is the Transmission Control Protocol (TCP). A TCP connection is established, data is transferred in a reliable and ordered manner, and the connection is terminated after the data exchange.
Connection-Less: An example of a connection-less service is the User Datagram Protocol (UDP). UDP sends data packets without establishing a connection, making it faster but less reliable compared to TCP.
Step-by-Step Implementation Guide:
Connection-Oriented:
1. Create a TCP socket using a server and client.
2. Establish a connection between the server and client using the socket.
3. Send data from the client to the server and vice versa.
4. Close the connection after the data exchange is complete.
Connection-Less:
1. Create a UDP socket using a server and client.
2. Send data packets from the client to the server without establishing a connection.
3. Handle packet loss and out-of-order arrival at the application level if needed.
Working Code Snippets:
Connection-Oriented (Python):
import socket
Server
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 9999))
server_socket.listen()
Client
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect(('localhost', 9999))
Connection-Less (Python):
import socket
Server
server_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
server_socket.bind(('localhost', 9999))
Client
client_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
client_socket.sendto(b'Hello, UDP!', ('localhost', 9999))
Best Practices and Optimization Tips:
- Connection-oriented services are best suited for applications requiring reliable and ordered data delivery.
- Connection-less services are more efficient for real-time applications or scenarios where occasional data loss is acceptable.
- Use connection-oriented protocols for critical data exchanges and connection-less protocols for streaming or broadcasting data.
Common Pitfalls and Solutions:
Pitfall: Overhead associated with connection-oriented protocols can impact performance.
Solution: Optimize TCP connections by reusing sockets and minimizing unnecessary data transfers.
Pitfall: Data loss in connection-less protocols can lead to incomplete transmissions.
Solution: Implement mechanisms like checksums or application-level acknowledgments to ensure data integrity in UDP transmissions.
Frequently Asked Questions: Connection-Oriented vs Connection-Less
What is the difference between connection-oriented and connectionless services?
In a connection-oriented service, the system establishes a dedicated communication path between two devices before transferring any data, ensuring orderly and error-free delivery. In contrast, a connectionless service sends each data packet independently without establishing a prior connection, providing no guarantees on delivery or order.
When should I choose a connection-oriented service?
You should choose a connection-oriented service when data integrity and reliability are crucial, such as when streaming multimedia content or transferring large files. Connection-oriented services guarantee data delivery and ensure that packets arrive in the correct order.
When is a connectionless service more suitable?
A connectionless service is more suitable when real-time communication is required or when the overhead of establishing and maintaining connections is undesirable. Applications like video conferencing or online gaming often favor connectionless services to reduce latency and improve responsiveness.
Can a single system support both connection-oriented and connectionless services simultaneously?
Yes, a single system can support both connection-oriented and connectionless services simultaneously. This flexibility allows applications to choose the appropriate communication mode based on their specific requirements, optimizing performance and efficiency.
Are connection-oriented services more secure than connectionless services?
While connection-oriented services offer inherent security benefits due to the established connection and data integrity checks, connectionless services can also be secured through encryption and other mechanisms. The level of security ultimately depends on the implementation of each service and additional security measures applied.
Conclusion
In conclusion, the choice between connection-oriented and connectionless services depends on the specific requirements and priorities of the system or application in question. Connection-oriented services establish a dedicated connection before data transfer, ensuring reliability and order, while connectionless services offer speed and efficiency with minimal overhead.
Key differences lie in the establishment of connections, reliability, sequencing, and overhead costs. Connection-oriented services are preferable for applications that prioritize data integrity and order, such as file transfer or streaming services. On the other hand, connectionless services are more suitable for real-time communication, gaming, or situations where speed is paramount.
Evaluate factors such as the nature of the data, required latency, network conditions, and scalability needs when choosing between the two. Choose connection-oriented services for mission-critical applications that require data integrity. Opt for connectionless services for time-sensitive applications that prioritize speed.
Ultimately, the best choice depends on striking a balance between reliability, speed, and overhead based on the specific requirements of your system. Assessing these decision-making criteria will help you determine whether a connection-oriented or connectionless service works best for your unique needs.