Hardware
Hardware refers to the physical components of a computer system that can be touched and seen. It includes devices such as the CPU, RAM, hard drive, motherboard, and peripherals like keyboards and monitors.
Example:
An example of hardware is a computer’s central processing unit (CPU), which executes instructions from software programs.
Advantages of Hardware:
- Fast processing speeds
- Direct interaction with physical world
- Reliable and stable
Disadvantages of Hardware:
- Expensive to manufacture
- Difficult to upgrade
- Physical limitations
Technical Characteristics:
- Physical components
- Requires power source
- Fixed and tangible
Use Cases and Applications:
- Embedded systems
- Industrial automation
- Consumer electronics
Software
Software refers to programs and applications that run on a computer system. It includes operating systems, applications, utilities, and programming languages that enable hardware to perform specific tasks.
Example:
An example of software is the Microsoft Windows operating system that manages hardware resources and provides a user interface.
Advantages of Software:
- Flexibility and scalability
- Easy to upgrade and modify
- Cost-effective compared to hardware
Disadvantages of Software:
- Prone to bugs and vulnerabilities
- Dependent on hardware for execution
- Compatibility issues
Technical Characteristics:
- Instructions and code
- Requires an operating environment
- Virtual and intangible
Use Cases and Applications:
- Enterprise software
- Mobile applications
- Video games
Key Differences b/w Hardware and Software
Hardware | Software |
---|---|
Physical components that can be touched and seen | Programs and data that can only be seen and not touched physically |
Requires installation, assembly, and physical configuration | Installed on hardware and configured through interfaces |
Has finite lifespan due to wear and tear | Can be updated and upgraded without physical replacement |
Impacted by environmental factors like temperature and humidity | Operates independently of environmental conditions |
Typically more expensive to replace or upgrade | Upgrades and updates can be relatively cost-effective |
Often requires physical maintenance and repairs | Can be debugged and updated remotely |
Performance is limited by physical capabilities | Performance can be optimized through code and algorithms |
May require physical space and infrastructure | Can be stored and accessed digitally |
Interactions are based on electrical signals and physical connections | Interactions are based on logical operations and code execution |
Usually has specific form factors and sizes | Can be scaled and adapted to different devices and platforms |
May have limited flexibility in terms of functionality | Can be customized and personalized through programming |
Typically requires specialized knowledge for physical handling | May require coding skills for customization and development |
Examples include CPUs, GPUs, motherboards, and physical peripherals | Examples include operating systems, applications, and utilities |
Primary focus is on processing and executing instructions | Primary focus is on providing functionality and features |
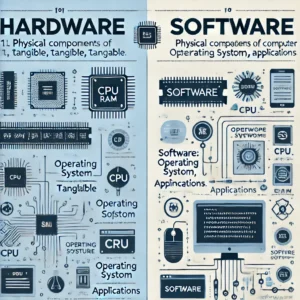
Practical Implementation
Hardware and software are essential components of computing systems, each with distinct characteristics and functionalities. In this guide, we will delve into practical implementation examples, working code snippets, step-by-step guides, best practices, and common pitfalls related to hardware and software.
Hardware Implementation Examples
Hardware refers to physical components of a computer system. Let’s consider a practical example of implementing hardware by building a basic electronic circuit using an Arduino board.
// Arduino LED Blink Example
const int LED_PIN = 13;
void setup() {
pinMode(LED_PIN, OUTPUT);
}
void loop() {
digitalWrite(LED_PIN, HIGH);
delay(1000);
digitalWrite(LED_PIN, LOW);
delay(1000);
}
Software Implementation Examples
Software comprises programs, scripts, and applications that run on hardware. Let’s illustrate software implementation through a simple Python script to calculate the factorial of a number.
Python Factorial Calculation
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
number = 5
result = factorial(number)
print(f'The factorial of {number} is {result}')
Step-by-Step Implementation Guide
1. Determine the hardware components needed for the project.
2. Assemble the hardware components according to the circuit diagram.
3. Write the code for the hardware functionality.
4. Upload the code to the Arduino board.
5. Test the hardware implementation and observe the output.
Best Practices and Optimization Tips
- For hardware, follow proper circuit design principles to ensure reliability.
- For software, optimize code by minimizing redundant operations and improving algorithm efficiency.
Common Pitfalls and Solutions
- Pitfall: In hardware projects, incorrect wiring can lead to malfunctioning.
- Solution: Double-check the connections and refer to the circuit diagram.
- Pitfall: In software development, inefficient algorithms can cause performance issues.
- Solution: Refactor the code to use more efficient algorithms or data structures.
Frequently Asked Questions
What is the primary difference between hardware and software?
Hardware refers to the physical components of a computer system, such as the processor, memory, and hard drive. Software, on the other hand, consists of programs and applications that run on the hardware to enable specific functions.
How does hardware differ from software in terms of permanence?
Hardware is tangible and physical, meaning it remains constant unless physically altered or upgraded. In contrast, software is intangible and can be easily modified, updated, or replaced without changing the physical components of the system.
Can hardware function without software?
While hardware is essential for a computer system to operate, it requires software to perform specific tasks. Without software, hardware remains idle and cannot execute any functions or processes.
How do hardware and software interact to enable computing tasks?
Hardware provides the physical infrastructure and processing power, while software instructs the hardware on how to perform tasks and processes data. The two work together in a symbiotic relationship to enable the functionality of a computer system.
Which is more susceptible to damage: hardware or software?
Hardware is typically more susceptible to physical damage, such as malfunctions due to overheating, electrical issues, or mechanical failures. Software, on the other hand, is prone to corruption from viruses, malware, or improper installation but can often be restored or replaced more easily than damaged hardware.
Conclusion
In conclusion, the comparison between hardware and software unveils crucial variances that shape the foundation of computing systems. Hardware constitutes the physical components of a device, while software comprises the intangible programs that enable functionality. Key differences lie in their tangibility, flexibility, and susceptibility to damage or corruption.
When deciding between hardware and software solutions, it is essential to consider factors such as cost, scalability, customization, and maintenance requirements. Hardware investments are typically more substantial upfront but offer long-term reliability and performance. On the other hand, software solutions provide greater flexibility, easier updates, and customization options.
To make an informed decision, organizations must assess their specific needs, budget constraints, technical expertise, and future growth plans. Prioritize reliability, performance, and compatibility when opting for hardware solutions, while emphasizing flexibility, ease of customization, and scalability for software solutions.
By carefully evaluating these decision-making criteria, businesses can choose the most suitable mix of hardware and software solutions to optimize their operations, enhance productivity, and achieve their strategic goals in the ever-evolving technological landscape.