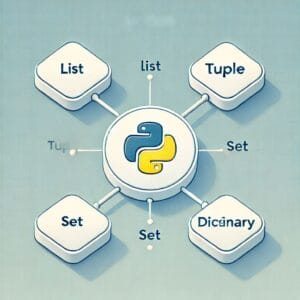
List
A list in Python is a collection of elements that is ordered and mutable. Lists are created using square brackets [] and can hold elements of different data types.
Example:
my_list = [1, 'apple', True] print(my_list)
Advantages:
- Mutable – Elements can be added, removed, or modified.
- Ordered – Elements maintain their order in the list.
Disadvantages:
- Slower performance for operations like insertion and deletion compared to sets and dictionaries.
Use Cases and Applications:
- Storing collections of similar items.
- Iterating over elements.
Tuple
A tuple in Python is a collection of elements that is ordered and immutable. Tuples are created using parentheses () and can also hold elements of different data types.
Example:
my_tuple = (1, 'banana', False) print(my_tuple)
Advantages:
- Immutable – Elements cannot be changed after creation.
- Faster access time compared to lists for read-only operations.
Disadvantages:
- Cannot be modified once created.
Use Cases and Applications:
- Used for fixed collections of elements where immutability is desired.
- Returning multiple values from a function.
Set
A set in Python is a collection of unique elements that is unordered and mutable. Sets are created using curly braces {} or the set() function.
Example:
my_set = {1, 2, 3} print(my_set)
Advantages:
- Contains unique elements only, eliminating duplicates.
- Fast membership testing and operations like intersection and union.
Disadvantages:
- Not ordered – Elements are not stored in a specific order.
Use Cases and Applications:
- Removing duplicates from a list.
- Checking for membership or common elements between sets.
Dictionary
You create dictionaries in Python using curly braces {}
and colons :
to define key-value pairs.
They let you store unordered and mutable collections of data.
Example:
my_dict = {'key1': 'value1', 'key2': 2} print(my_dict)
Advantages:
- Fast lookups based on keys.
- Key-value pair structure allows for easy data retrieval.
Disadvantages:
- Not ordered – No guarantee on the order of key-value pairs.
Use Cases and Applications:
- Storing data with a key for easy retrieval.
- Mapping unique identifiers to values for quick access.
Key Differences: List, Tuple, Set, and Dictionary
List | Tuple | Set | Dictionary |
---|---|---|---|
Mutable | Immutable | Mutable | Mutable |
Defined with square brackets [] | Defined with parentheses () | Defined with curly braces {} | Defined with curly braces {} (key-value pairs) |
Supports item assignment and deletion | Does not support item assignment or deletion | Supports item addition and deletion | Supports item addition, deletion, and modification of values |
Has more built-in methods like append(), extend() | Has fewer built-in methods compared to list | Has methods like add(), remove(), and discard() | Has methods like keys(), values(), items(), and get() |
Can contain duplicate elements | Can contain duplicate elements | Cannot contain duplicate elements | Keys must be unique, but values can be duplicated |
Ordered collection | Ordered collection | Unordered collection | Ordered collection (as of Python 3.7) |
Can be used as keys in dictionaries | Can be used as keys in dictionaries if they contain only hashable elements | Cannot be used as keys in dictionaries | Keys are used as identifiers in dictionaries |
Slower iteration compared to tuples | Faster iteration than lists | Faster iteration than lists | Moderately fast iteration depending on the number of keys |
Can store mixed data types | Can store mixed data types | Can store mixed data types | Can store mixed data types (keys and values) |
More memory consumption due to mutability | Less memory consumption due to immutability | More memory-efficient compared to lists | Memory-efficient, especially for large datasets |
Commonly used for variable-sized collections | Preferred for fixed-size collections or when immutability is required | Preferred for collections where uniqueness is important | Used to store key-value pairs for efficient data lookup |
Can contain any data type | Can contain any data type | Can contain any data type | Keys must be hashable, values can be any data type |
Can contain nested lists | Can contain nested tuples | Can contain nested sets or frozensets | Can contain nested dictionaries |
More flexible in terms of operations | More secure in terms of data integrity | Efficient for membership tests | Efficient for quick lookups and data association |
Commonly used for data that needs frequent updates | Used for data that should not change over time | Used for data that must have unique elements | Commonly used for key-value mappings |
Practical Implementation
Lists in Python
Lists are versatile data structures in Python that can hold heterogeneous elements.
Practical Implementation Example:
Let’s create a list of fruits and print each fruit:
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
Best Practices and Optimization Tips:
Use list comprehension for concise and efficient code:
squared_nums = [num2 for num in range(1, 6)]
Common Pitfalls and Solutions:
A common pitfall is modifying a list while iterating over it. To avoid this, create a copy of the list:
numbers = [1, 2, 3]
for num in numbers[:]:
numbers.append(num * 2)
Tuples in Python
Tuples are immutable sequences in Python, typically used to represent fixed collections of items.
Practical Implementation Example:
Creating a tuple of coordinates:
coordinates = (10, 20)
x, y = coordinates
print(f'x: {x}, y: {y}')
Best Practices and Optimization Tips:
Use tuples as keys in dictionaries for efficient lookups:
point = (3, 4)
distances = {(0, 0): 0, (1, 1): 1}
distance_to_origin = distances.get(point, -1)
Common Pitfalls and Solutions:
Attempting to modify a tuple will result in an error. If mutability is required, consider using a list.
Sets in Python
Use sets in Python when you need to store unique elements, test for membership, or eliminate duplicates without caring about order.
Practical Implementation Example:
Creating a set of unique letters in a word:
word = 'hello'
unique_letters = set(word)
print(unique_letters)
Best Practices and Optimization Tips:
Use set operations like intersection, union, and difference for efficient manipulation of data:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
intersection = set1 & set2
Common Pitfalls and Solutions:
Accidentally mutating a set during iteration can lead to unexpected behavior. To prevent this, operate on a copy of the set.
Dictionaries in Python
Dictionaries are key-value pairs that allow for fast lookups and mappings between items.
Practical Implementation Example:
Creating a dictionary of phone contacts:
contacts = {'Alice': '555-1234', 'Bob': '555-5678'}
print(contacts['Alice'])
Best Practices and Optimization Tips:
Use dictionary comprehensions for concise creation of dictionaries:
squared_dict = {num: num2 for num in range(1, 6)}
Common Pitfalls and Solutions:
Trying to access a non-existent key in a dictionary can raise a KeyError. To handle this gracefully, use the get method with a default value.
FAQs: List, Tuple, Set, and Dictionary
What are the key distinctions between lists, tuples, sets, and dictionaries in
Python?
Lists, tuples, sets, and dictionaries are all data structures in Python, but they serve different purposes.
You can change, add, or remove items in a list, which keeps them in order.
Tuples work like lists, but you can’t change their elements after you create them.
Sets store unique elements without duplicates and don’t maintain any specific order.
Dictionaries are key-value pairs where each key is unique, allowing efficient lookup of values.
How can I decide which data structure to use among lists, tuples, sets, and dictionaries?
Use a list to keep an ordered collection you can change.
Pick a tuple when your values stay the same.
Go with a set to store unique items without worrying about order.
Choose a dictionary to link keys to values and retrieve data quickly.
Can data structures in Python be nested within each other?
Yes, you can nest data structures within each other in Python.
For example, you can have a list that contains tuples, a dictionary with lists as values, or even a set of dictionaries.
This allows for more complex data structures to represent real-world relationships.
How do I access elements within lists, tuples, sets, and dictionaries?
To access elements in a list or tuple, you can use indexing (e.g., list[0] or tuple[1]).
You can iterate over the elements in a set, but don’t expect them to follow any order.
In dictionaries, you can access values by their keys (e.g., dict[‘key’]).
Are there any performance differences between lists, tuples, sets, and dictionaries?
Yes, there are performance differences among these data structures.
You can iterate over lists quickly, and they allow duplicate elements.
Tuples are faster than lists for iteration but are immutable.
Sets offer fast membership checks for unique elements.
Dictionaries provide fast lookup times due to their key-value mapping.
Conclusion
In conclusion, understanding the distinctions between Python’s list, tuple, set, and dictionary is essential for efficient and effective programming. Lists are mutable and ordered collections, suitable for storing and manipulating data that may change over time. Tuples, on the other hand, are immutable and ordered, making them ideal for protecting data integrity. Use sets when you need distinct values, since they don’t keep order and only store unique elements. Lastly, dictionaries are key-value pairs that provide fast data retrieval based on keys.
When selecting a data structure, consider the specific requirements of your program. If you need to work with a collection of items that may change frequently, opt for a list. For data that should remain constant, use a tuple to prevent accidental modifications. Use sets when dealing with unique elements and require efficient membership testing and operations like intersection and union. Dictionaries are perfect for scenarios where fast data retrieval based on keys is crucial.
Decision-making criteria should include factors such as data mutability, order preservation, uniqueness of elements, and key-value pairing requirements. By carefully evaluating these aspects, you can choose the most suitable data structure that aligns with your programming needs, leading to more robust and efficient code.
https://diffstudy.com/python-lists-vs-tuples-key-differences-you-should-know/