SQL Databases
SQL databases are relational databases that use structured query language (SQL) for defining and manipulating data. Here are some key points:
- Structured Data: SQL databases store data in tables with predefined schema.
- ACID Compliance: They ensure Atomicity, Consistency, Isolation, and Durability.
- Vertical scalability is achieved by increasing the power of the hardware.
- Examples: MySQL, PostgreSQL, Oracle.
Advantages:
- Strong Consistency: Data is consistent across all operations.
- Transaction Support: Supports complex transactions.
- ACID Properties: Ensures data integrity.
Disadvantages:
- Scaling Challenges: Limited scalability for large-scale applications.
- Complexity: Structured data model can be limiting for certain use cases.
NoSQL Databases
NoSQL databases handle large volumes of unstructured data and provide flexibility due to their non-relational design.
Key points include:
- Flexible Schema: NoSQL databases can handle unstructured and semi-structured data.
- Horizontal scalability is achieved by adding more servers to the database.
- Examples: MongoDB, Cassandra, Redis.
Advantages:
- Scalability: Easily scales horizontally to handle large amounts of data.
- Flexibility: Can handle diverse data types and structures.
- Performance: High performance for read and write operations.
Disadvantages:
- Consistency: Eventual consistency may lead to data conflicts.
- Lack of Transactions: Limited support for complex transactions.
Use Cases and Applications
SQL databases fit traditional applications with well-defined data structures and a need for consistency. Examples include financial systems, e-commerce platforms, and CRM applications.
NoSQL databases support applications that need flexible data models, high scalability, and fast performance. They are commonly used in social networks, IoT applications, real-time analytics, and content management systems.
Key Differences between SQL and NoSQL
SQL Databases | NoSQL Databases |
---|---|
Structured Query Language databases | Non-relational databases |
SQL databases are table-based | NoSQL databases can be document-based, key-value pairs, graph databases, etc. |
SQL databases are best suited for complex queries and transactions | NoSQL databases are better for hierarchical data storage |
SQL databases use predefined schema | NoSQL databases have dynamic schema for unstructured data |
ACID properties are guaranteed in SQL databases | NoSQL databases sacrifice some ACID properties for scalability and performance |
SQL databases are vertically scalable | NoSQL databases are horizontally scalable |
Joins are supported in SQL databases | NoSQL databases have limited or no support for joins |
SQL databases are well suited for complex queries | NoSQL databases are optimized for read and write operations |
Transactions are supported in SQL databases | NoSQL databases may not support ACID transactions across multiple documents/collections |
SQL databases are mature and have been around for a longer time | NoSQL databases are relatively newer and more flexible |
SQL databases are generally not horizontally scalable | NoSQL databases are designed for distributed data stores that can scale horizontally |
SQL databases are good for complex queries | NoSQL databases are not as good for complex queries |
Consistency is usually maintained more strictly in SQL databases | NoSQL databases may offer eventual consistency |
SQL databases are often used in traditional RDBMS setups | NoSQL databases are commonly used in modern cloud-based or big data applications |
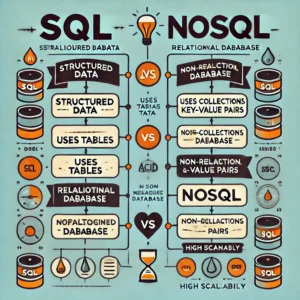
Practical Implementation
SQL (Structured Query Language) and NoSQL (Not Only SQL) are two types of database management systems that differ in their structure, flexibility, and scalability. Understanding the differences between SQL and NoSQL databases is crucial for choosing the right system for your specific use case.
SQL Example
// Connect to a MySQL database
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "myDB";
// Create a connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Execute a simple SQL query
$sql = "SELECT * FROM users";
$result = $conn->query($sql);
// Fetch and display results
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo "Name: " . $row["name"]. " - Email: " . $row["email"]. "
";
}
} else {
echo "0 results";
}
// Close the connection
$conn->close();
NoSQL Example
// Connect to a MongoDB database
$client = new MongoDB\Client("mongodb://localhost:27017");
// Select a database and a collection
$collection = $client->mydb->users;
// Insert a document into the collection
$result = $collection->insertOne([
'name' => 'John Doe',
'email' => 'johndoe@example.com'
]);
// Find a document in the collection
$user = $collection->findOne(['_id' => $result->getInsertedId()]);
// Print the user details
var_dump($user);
Step-by-Step Implementation Guide
- Define Your Data Model: Determine the structure of your data and relationships between entities.
- Choose the Right Database Type: Select SQL for structured data with complex relationships or NoSQL for unstructured or rapidly changing data.
- Design the Database Schema: Create tables, collections, or key-value pairs based on your data model.
- Implement Data Operations: Write queries or commands to insert, update, retrieve, and delete data.
- Optimize Queries: Index columns, use appropriate joins, and normalize data to improve query performance.
Best Practices and Optimization Tips
- SQL: Use indexes on frequently queried columns, normalize data to reduce redundancy, and optimize complex queries.
- NoSQL: Denormalize data for faster reads, distribute data across multiple nodes for scalability, and use appropriate data models for efficient queries.
Common Pitfalls and Solutions
- SQL Pitfall: Over-reliance on JOIN operations can lead to performance issues, especially with large datasets. Solution: Limit unnecessary joins and denormalize data where necessary.
- NoSQL Pitfall: Lack of transaction support can result in data inconsistencies. Solution: Use atomic operations and implement application-level transactions.
Frequently Asked Questions
What is the main difference between SQL and NoSQL databases?
SQL databases are relational databases that store data in tables with predefined schemas, while NoSQL databases are non-relational databases that store data in flexible, schema-less formats like key-value pairs, documents, or graphs.
How does data consistency differ between SQL and NoSQL databases?
SQL databases typically ensure strong consistency, processing all transactions reliably. In contrast, NoSQL databases often sacrifice some level of consistency for scalability and performance, offering eventual consistency where data may take time to propagate.
Can you explain the scalability differences between SQL and NoSQL databases?
SQL databases traditionally scale vertically by adding more resources to a single server, while NoSQL databases are designed to scale horizontally, distributing data across multiple servers to handle large volumes of data and traffic more effectively.
How do SQL and NoSQL databases handle complex queries?
SQL databases optimize complex queries involving multiple tables and relationships through the use of structured query language (SQL). On the other hand, NoSQL databases may struggle with complex queries as they prioritize high-speed data retrieval and simple data models.
What are some use cases where SQL databases are preferred over NoSQL databases?
Applications that require ACID transactions, strict data consistency, and complex queries, such as financial systems, e-commerce platforms, and traditional enterprise applications, often prefer SQL databases due to their emphasis on data integrity.
Conclusion
In conclusion, the fundamental differences between SQL and NoSQL databases lie in their data structure, scalability, and flexibility. SQL databases offer a structured, relational model suitable for complex queries and transactions, making them ideal for applications requiring ACID compliance and strict data consistency. On the other hand, NoSQL databases provide a flexible, schema-less design that enables scalability and performance for distributed systems and applications with large volumes of unstructured data.
When choosing between SQL and NoSQL databases, it is essential to consider the specific requirements of your application. If your application demands complex queries, structured data, and transactional support, SQL databases like MySQL or PostgreSQL may be the preferred choice. Conversely, if your application requires horizontal scalability, flexibility in data modeling, and high availability, NoSQL databases such as MongoDB or Cassandra may be more suitable.
Decision-making criteria should be based on factors such as data complexity, scalability needs, consistency requirements, and development flexibility. It is crucial to evaluate the trade-offs between data consistency and performance, as well as consider future growth and adaptability of your application.
Ultimately, the choice between SQL and NoSQL databases should align with your application’s specific needs and long-term goals to ensure optimal performance and scalability.