Java’s polymorphism plays a key role in object-oriented programming. The two main types, compile-time and run-time polymorphism, differ in their implementation. Understanding these differences is crucial for mastering Java programming. In this article, we explore what sets compile-time vs. run-time polymorphism apart.
Compile-time Polymorphism
Compile-time polymorphism, also known as static polymorphism, is achieved using method overloading and operator overloading. It is resolved during compile time based on the method signature.
Example of Compile-time Polymorphism:
public class Calculator {
// Method overloading
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
}
Advantages:
- Efficient execution as the method is bound during compilation
- Compile-time error detection if method resolution fails
Disadvantages:
- Less flexibility compared to run-time polymorphism
- Requires method overloading or operator overloading
Run-time Polymorphism
Run-time polymorphism, also known as dynamic polymorphism, is achieved using method overriding. It is resolved during runtime based on the actual object type.
Example of Run-time Polymorphism:
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
void sound() {
System.out.println("Dog barks");
}
}
class Cat extends Animal {
void sound() {
System.out.println("Cat meows");
}
}
public class Main {
public static void main(String[] args) {
Animal a = new Dog();
a.sound(); // Output: Dog barks
a = new Cat();
a.sound(); // Output: Cat meows
}
}
Advantages:
- Enhanced flexibility and extensibility
- Enables method overriding for specific behavior in subclasses
Disadvantages:
- Slightly slower performance compared to compile-time polymorphism
- May introduce complexity in understanding code flow
Technical Characteristics
Compile-time polymorphism is resolved at compile time based on the method signature, while run-time polymorphism is resolved at runtime based on the actual object type.
Use Cases and Applications
Compile-time polymorphism is suitable for scenarios where method overloading can provide different behavior based on parameter types. Run-time polymorphism is useful when different subclasses need to exhibit specific behaviors through method overriding.
Key Differences and Analysis
Compile-time Polymorphism | Run-time Polymorphism |
---|---|
Resolved during compilation | Resolved during runtime |
Determined by the compiler based on reference type | Determined by the JVM based on the actual object type |
Also known as static binding or early binding | Also known as dynamic binding or late binding |
Method overloading is an example | Method overriding is an example |
Requires method signature to be resolved at compile time | Requires actual method implementation to be resolved at runtime |
Efficient in terms of performance | May incur a slight performance overhead due to dynamic dispatch |
Changes to the method signature necessitate recompilation | No need for recompilation if method signatures remain unchanged |
Commonly achieved using method overloading | Commonly achieved using method overriding with inheritance |
Examples include function overloading, operator overloading | Examples include virtual functions, abstract classes, interfaces |
Decision based on reference type leads to early error detection | Decision based on object type may lead to run-time errors |
More predictable and easier to trace during development | May require tracing through runtime behavior for complete understanding |
Static binding requires less runtime overhead | Dynamic binding may lead to more flexibility but with added runtime cost |
Common in method calls within the same class | Common in method calls across different classes in an inheritance hierarchy |
Compile-time failures may indicate issues in the code logic | Run-time failures might be harder to predict and trace back |
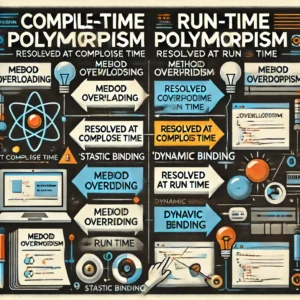
Practical Implementation
Detailed Practical Implementation Examples
Compile-time Polymorphism
Compile-time polymorphism in Java is achieved through method overloading.
public class OverloadingExample {
public void display(int num) {
System.out.println("Number: " + num);
}
public void display(String text) {
System.out.println("Text: " + text);
}
}
Run-time Polymorphism
Run-time polymorphism in Java is achieved through method overriding.
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
void sound() {
System.out.println("Dog barks");
}
}
Working Code Snippets
Below are working code snippets demonstrating compile-time and run-time polymorphism:
public class Main {
public static void main(String[] args) {
// Compile-time polymorphism
OverloadingExample overloadingExample = new OverloadingExample();
overloadingExample.display(10);
overloadingExample.display("Hello");
// Run-time polymorphism
Animal animal = new Dog();
animal.sound();
}
}
Step-by-step Implementation Guide
- Create classes and define methods for compile-time and run-time polymorphism.
- Implement method overloading for compile-time polymorphism.
- Implement method overriding for run-time polymorphism.
- Create instances of the classes and call the methods to observe polymorphic behavior.
Best Practices and Optimization Tips
- Use method overloading sparingly and ensure clear distinction between overloaded methods.
- For run-time polymorphism, follow Liskov Substitution Principle to maintain code flexibility.
- Avoid excessive method overriding to prevent complexity and confusion.
Common Pitfalls and Solutions
- Pitfall: Confusing method overloading with overriding.
- Solution: Understand the differences between overloading and overriding to use them appropriately.
Frequently Asked Questions
What is compile-time polymorphism in Java?
Compile-time polymorphism in Java refers to the method overloading feature where multiple methods can have the same name but with different parameters or return types. The compiler determines which method to call based on the method signature during the compilation phase.
How does run-time polymorphism differ from compile-time polymorphism in Java?
Run-time polymorphism in Java is achieved through method overriding, where a subclass provides a specific implementation of a method that is already defined in its superclass. The decision on which method to call is made at runtime based on the actual object type rather than the reference type.
Can you provide an example of compile-time polymorphism in Java?
For example, consider a class with two methods: calculateArea(int side) and calculateArea(int length, int breadth). Both methods have the same name but different parameter lists. The compiler determines which method to call based on the number and types of arguments passed when the method is invoked.
How is run-time polymorphism implemented in Java?
Run-time polymorphism in Java is implemented through method overriding, where a subclass provides its own implementation of a method defined in its superclass. To achieve run-time polymorphism, you need to use inheritance and ensure that the subclass overrides the superclass method with the same signature.
What are the key benefits of using polymorphism in Java?
Using polymorphism in Java allows for code reusability, flexibility, and extensibility. It enables you to write more generic and maintainable code by allowing different implementations to be used interchangeably based on the context in which they are called.
Conclusion
In conclusion, understanding the distinctions between Java’s compile-time and run-time polymorphism is crucial for Java developers to write efficient and maintainable code. Compile-time polymorphism, achieved through method overloading and overriding, is resolved during compilation, offering better performance but less flexibility. On the other hand, run-time polymorphism, realized through inheritance and interfaces, provides greater flexibility at the cost of slightly reduced performance.
When deciding between compile-time and run-time polymorphism in Java, developers should consider their specific requirements and objectives. For situations where performance is critical and method signatures differ, compile-time polymorphism is recommended. Conversely, if flexibility and dynamic behavior are prioritized, run-time polymorphism is the preferable choice.
Ultimately, the decision-making criteria should be based on the project’s performance needs, design complexity, and future scalability. By carefully evaluating these factors, developers can leverage the strengths of each type of polymorphism to optimize their Java applications effectively.