Depth-First Search (DFS) vs. Breadth-First Search (BFS)
Graph traversal algorithms are crucial in computer science, helping to explore nodes and edges in graphs efficiently. Two primary methods for this are Depth-First Search (DFS) and Breadth-First Search (BFS). Understanding the differences between these algorithms is essential for solving various computational problems.
Depth-First Search (DFS)
Depth-First Search (DFS) is an algorithm that starts at a root node and explores as far down each branch as possible before backtracking. This deep exploration method makes DFS suitable for scenarios requiring comprehensive path exploration.
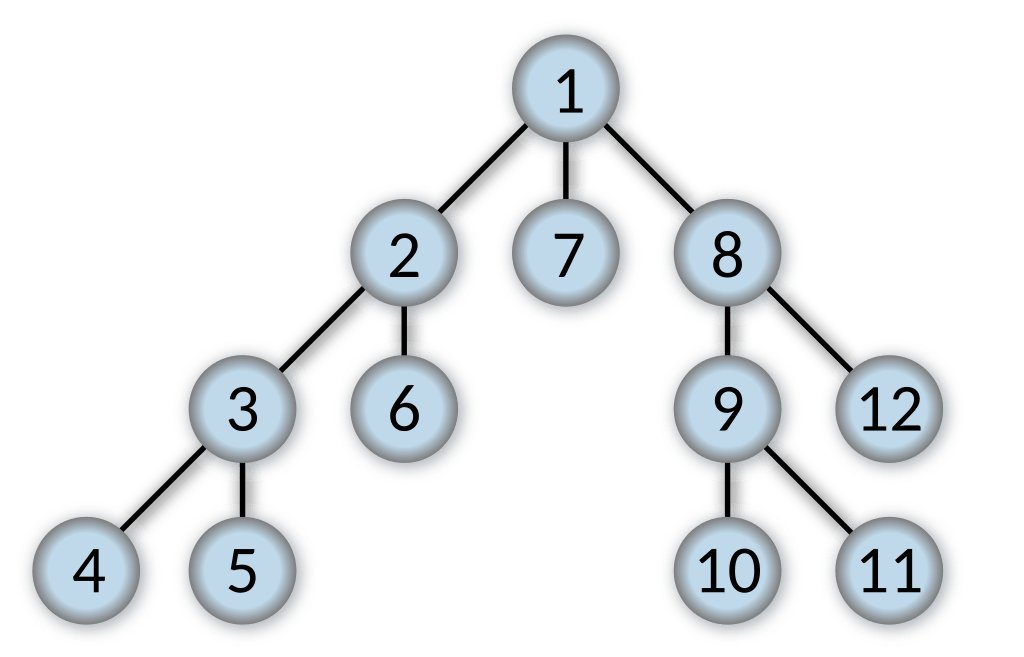
Characteristics of DFS:
Strategy: DFS dives deep into the graph, visiting each child node before moving to sibling nodes.
Implementation: Typically implemented using a stack or through recursion.
Space Complexity: In the worst case, the space complexity is O(V), where V is the number of vertices, due to the storage of the recursion stack or explicit stack.
Time Complexity: DFS has a time complexity of O(V + E), where V is vertices and E is edges.
Use Cases: Useful for solving puzzles, exploring mazes, and finding strongly connected components in graphs.
Breadth-First Search (BFS)
Breadth-First Search (BFS) is an algorithm that starts at the root node and explores all its neighbours before moving to the next level of nodes. This level-wise exploration ensures that all nodes at a given depth are visited before any deeper nodes.
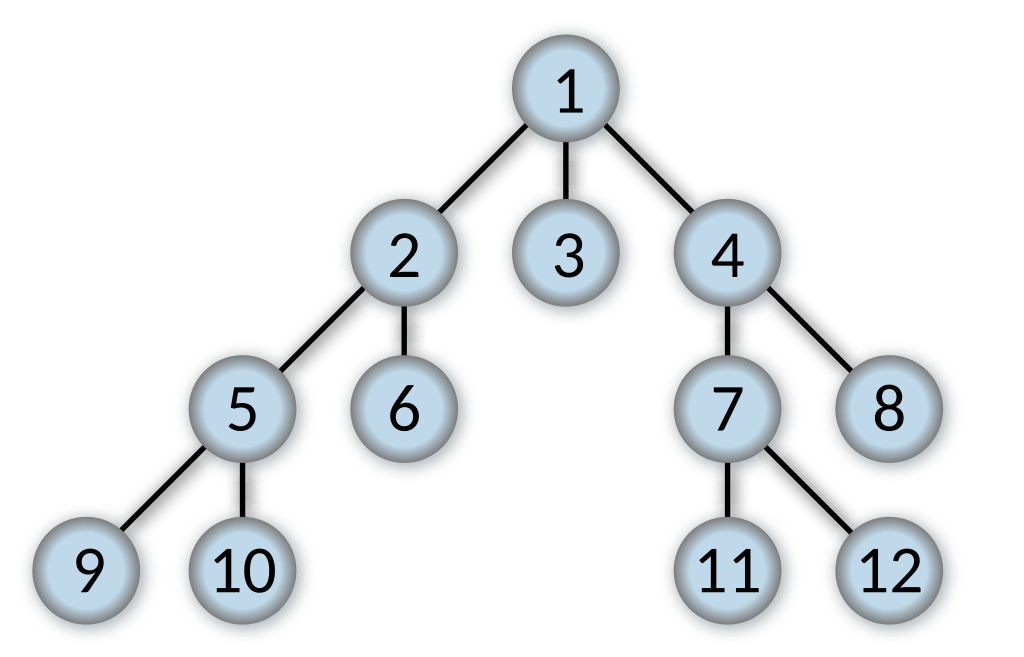
Characteristics of BFS:
Strategy: BFS explores all nodes at the present depth level before moving to nodes at the next depth level.
Implementation: Implemented using a queue data structure.
Space Complexity: O(V), where V is the number of vertices, due to the queue used for storing nodes.
Time Complexity: The time complexity is O(V + E), where V is vertices and E is edges.
Use Cases: Ideal for finding the shortest path in unweighted graphs, level-order traversal in trees, and applications in social networks and web crawling.
Key Differences Between DFS and BFS
Feature | Depth-First Search (DFS) | Breadth-First Search (BFS) |
---|---|---|
Strategy | Explores as far down a branch as possible before backtracking. | Explores all neighbors at the present depth level before moving to the next level. |
Implementation | Uses a stack or recursion. | Uses a queue. |
Space Complexity | O(V), where V is the number of vertices (due to stack or recursion stack). | O(V), where V is the number of vertices (due to the queue). |
Time Complexity | O(V + E), where V is vertices and E is edges. | O(V + E), where V is vertices and E is edges. |
Traversal Order | Deep in each branch before backtracking. | Level by level, visiting all nodes at the current depth before moving to the next. |
Use Cases | Solving puzzles, exploring mazes, topological sorting, finding strongly connected components. | Finding shortest paths in unweighted graphs, level-order traversal, social networking analysis. |
Shortest Path | Not typically used for finding shortest paths. | Used for finding shortest paths in unweighted graphs. |
Conclusion
Both DFS and BFS are powerful graph traversal algorithms, each with its unique strengths and applications. DFS is suitable for scenarios requiring deep exploration, while BFS excels in finding the shortest path and performing level-order traversal. Mastering these algorithms equips you with essential tools for solving a variety of computational problems.
FAQs on Depth-First Search (DFS) vs. Breadth-First Search (BFS)
Q 1. What is Depth-First Search (DFS)?
Depth-First Search (DFS) is an algorithm used to traverse or search through a graph or tree. It starts at a chosen node and explores as far as possible along each branch before backtracking. DFS can be implemented using a stack or through recursion, and it’s often used for tasks that require exploring every path or configuration in a structure.
Q 2. What is Breadth-First Search (BFS)?
Breadth-First Search (BFS) is a graph traversal algorithm that begins at a starting node and explores all of its neighbors before moving on to nodes at the next level of depth. BFS uses a queue to manage the nodes to be explored, making it effective for finding the shortest path in unweighted graphs and performing level-order traversal in trees.
Q 3. How do DFS and BFS differ in their traversal methods?
DFS: This method delves deeply into each branch of the graph, exploring all possible paths from the starting node before backtracking and moving to other branches.
BFS: This method explores all nodes at the present depth level before proceeding to nodes at the next level, ensuring a level-by-level exploration.
Q 4. What are the primary applications of DFS?
DFS is particularly useful for:
- Solving complex puzzles and mazes
- Conducting topological sorting
- Identifying strongly connected components in networks
- Analyzing network vulnerabilities and connectivity
Q 5. What are the main uses of BFS?
BFS is commonly used for:
- Finding the shortest path between nodes in an unweighted graph
- Performing level-order traversal of trees
- Implementing algorithms for social network analysis and web crawling
Q 6. What are the time and space complexities for DFS and BFS?
DFS:
Time Complexity: O(V + E), where V represents the number of vertices and E the number of edges
Space Complexity: O(V) due to the stack or recursion stack used
BFS:
Time Complexity: O(V + E), where V represents the number of vertices and E the number of edges
Space Complexity: O(V) because of the queue used to keep track of nodes
Q 7. Is DFS suitable for finding the shortest path?
DFS is not typically used for finding the shortest path in graphs, especially unweighted ones, because it does not guarantee the shortest path due to its depth-first nature. BFS is generally preferred for shortest path algorithms in such cases.
Q 8. What advantages does BFS offer over DFS?
BFS ensures that the shortest path is found in an unweighted graph.
It is more suitable for problems requiring exploration of nodes level by level, such as shortest path algorithms in social networks and other applications.
Q 9. Can DFS and BFS handle cyclic graphs?
Yes, both DFS and BFS can be applied to cyclic graphs. It is important to implement mechanisms to track visited nodes to prevent infinite loops during traversal.
Q 10. How are DFS and BFS typically implemented in code?
- DFS can be implemented using either a stack or recursion. The stack keeps track of nodes to visit, while recursion utilizes the call stack for traversal.
- BFS is implemented using a queue, which maintains the order of nodes to be explored as they are discovered.