Lists
A list is a versatile and mutable collection of items in Python. It is defined using square brackets [] and allows for elements to be added, removed, or modified.
Example:
my_list = [1, 2, 'hello', True]
Advantages of Lists:
- Mutable: Elements can be modified after creation.
- Dynamic: Size can be changed by adding or removing elements.
- Versatile: Supports various operations like slicing, concatenation, and more.
Disadvantages of Lists:
- Less Efficient: Lists consume more memory compared to tuples.
- Slower: Manipulating lists is slower than tuples due to mutability.
Tuples
A tuple is an immutable collection of items in Python. It is defined using parentheses () and once created, its elements cannot be changed.
Example:
my_tuple = (3, 4, 'world', False)
Advantages of Tuples:
- Immutable: Data integrity is ensured as elements cannot be changed accidentally.
- Faster Access: Tuples are more efficient for accessing elements.
- Safe Iteration: Prevents accidental modification during iteration.
Disadvantages of Tuples:
- Immutability: Once created, elements cannot be modified.
- Limited Operations: Limited support for operations compared to lists.
Technical Characteristics
Lists are mutable objects in Python, meaning you can change their elements. Tuples, however, are immutable objects, and you cannot modify their elements once created.
Use Cases and Applications
Use lists when flexibility and mutability are required, such as when storing dynamic data or frequently modifying elements. Opt for tuples when data integrity and immutability are crucial, like in scenarios where you need to prevent changes after creation.
Python Lists vs Tuples Key Differences
Lists | Tuples |
---|---|
Mutable | Immutable |
Defined with square brackets [ ] | Defined with parentheses ( ) |
Supports methods like append(), extend(), and remove() | Does not support methods that modify the tuple |
Elements can be changed or reassigned | Elements cannot be changed after creation |
Commonly used for dynamic data | Used for fixed data that shouldn’t change |
Used for sequences of items of the same type or related data | Used for heterogeneous data or to provide structure |
Slower when it comes to iteration compared to tuples | Faster for iteration compared to lists |
Occupies more memory due to flexibility | Occupies less memory due to immutability |
Can be used as keys in dictionaries | Cannot be used as keys in dictionaries |
Flexible for changes in size and content | Intended for fixed structures or read-only data |
Can be nested and store different types of data | Can also be nested but are more suitable for homogeneous data |
Commonly used when the number of elements may change | Used when the number of elements is fixed or known |
More error-prone since it allows modification after creation | Less error-prone due to immutability |
Often used for implementing stacks and queues | Useful for protecting data integrity and ensuring data consistency |
Operations like sorting can alter the original list | Operations do not alter the original tuple |
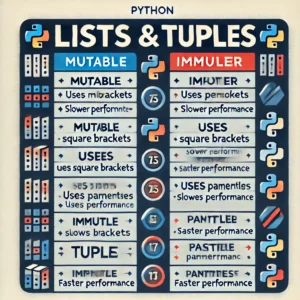
Practical Implementation
Lists and tuples are both common data structures in Python, but they have distinct characteristics. Let’s explore some practical examples to understand the differences:
Working Code Snippets:
List example
my_list = [1, 2, 3, 4, 5]
my_list.append(6)
print(my_list)
Tuple example
my_tuple = (1, 2, 3, 4, 5)
my_tuple.append(6) This will raise an error as tuples are immutable
print(my_tuple)
Step-by-Step Implementation Guide:
- Create a list using square brackets [] or a tuple using parentheses ().
- Use list methods like append(), extend(), remove(), etc., for lists.
- Access elements in a list using indexing.
- Tuples are immutable, so they cannot be modified once created.
Best Practices and Optimization Tips:
- Use lists when you need a collection of items that can be modified.
- Use tuples for data that should not change throughout the program execution.
- Consider using tuples for performance-critical code as they are slightly faster than lists.
Common Pitfalls and Solutions:
One common pitfall is trying to modify a tuple which will result in an error. To resolve this, always use lists when you need to alter the data structure during the program execution.
Frequently Asked Questions
What are the key differences between lists and tuples in Python?
Lists and tuples are both sequential data types in Python, but the main difference is that lists are mutable, while tuples are immutable. This means that elements in a list can be changed, added, or removed after the list is created, whereas elements in a tuple cannot be modified once the tuple is defined.
When should I use a list instead of a tuple?
Use a list when you have a collection of items that may need to be modified, reordered, or have elements added or removed. Lists are a good choice when you need a dynamic data structure. Tuples are best suited for situations where the data should not change, such as when representing fixed collections of items like coordinates or database records.
Can lists and tuples contain different types of elements?
Yes, both lists and tuples can contain elements of different data types. You can have a mix of integers, strings, floats, other lists, or even tuples within a single list or tuple in Python. This flexibility allows you to create versatile data structures to suit your needs.
What are some common operations that can be performed on lists and tuples?
Common operations on lists and tuples include accessing elements by index, slicing to extract subsets of elements, concatenating multiple lists or tuples, checking for membership using the ‘in’ operator, and iterating over the elements using loops like ‘for’ and ‘while’ loops.
How does the performance of lists differ from tuples?
Due to the immutability of tuples, they are generally faster and more memory-efficient than lists. Tuples have a fixed size and structure, which allows for quicker access to elements compared to lists. If you have a large collection of data that does not need to be modified, using tuples can lead to better performance in your Python programs.
Conclusion
In conclusion, understanding the contrast between lists and tuples in Python is essential for efficient programming. Lists are mutable, allowing for dynamic changes, while tuples are immutable, ensuring data integrity. Key differences lie in their usage based on the need for flexibility or data protection.
For optimal performance, consider using lists for scenarios where elements may change, and tuples for data that should remain constant. Decision-making criteria includes evaluating the nature of the data, the need for modification, and the level of data security required. By carefully considering these factors, developers can leverage the strengths of both lists and tuples to enhance code functionality and maintain data integrity in Python programming.
Exploring Python’s List, Tuple, Set, and Dictionary
[…] Python Lists vs Tuples: Key Differences You Should Know […]