Stack vs. Queue Data Structures
A stack is a linear data structure that follows the Last In First Out (LIFO) principle, where elements are added and removed from the same end known as the top. On the other hand, a queue is also a linear data structure but follows the First In First Out (FIFO) principle, where elements are added to the rear end and removed from the front end.
Example of a stack:
stack.push(1); stack.push(2); stack.push(3); stack.pop(); // Removes 3
Example of a queue:
queue.enqueue(1); queue.enqueue(2); queue.enqueue(3); queue.dequeue(); // Removes 1
Advantages and Disadvantages
Stack Advantages:
- Simple implementation
- Efficient for managing function calls, undo mechanisms
Stack Disadvantages:
- Not suitable for tasks requiring multiple access points
Queue Advantages:
- Efficient for managing resources in a networked environment
- Used in scheduling tasks and data processing
Queue Disadvantages:
- Slower than stack for some operations
Technical Characteristics
Stack and Queue data structures are typically implemented using arrays or linked lists. Both have operations like push/pop for stacks and enqueue/dequeue for queues.
Use Cases and Applications
Stack:
- Undo mechanisms in text editors
- Function call management
- Expression evaluation in compilers
Queue:
- Network packet scheduling
- Print job scheduling in printers
- Breadth-first search in graphs
Key Differences Stack vs Queue
Stack | Queue |
---|---|
Operates on Last-In-First-Out (LIFO) principle | Operates on First-In-First-Out (FIFO) principle |
Supports operations like push and pop | Supports operations like enqueue and dequeue |
Elements are accessed in reverse order of insertion | Elements are accessed in the same order as inserted |
Commonly implemented using arrays or linked lists | Commonly implemented using linked lists or circular buffers |
Allows access to only the top element | Allows access to both front and rear elements |
Used in applications like function call stack, undo operations | Used in applications like task scheduling, breadth-first search |
Can be inefficient for searching or accessing arbitrary elements | Supports efficient access to elements at both ends |
Stack overflow occurs when pushing elements beyond capacity | Queue overflow occurs when adding elements beyond capacity |
Has peek operation to view the top element without removal | Has front and rear operations to view elements without removal |
Supports depth-first search algorithms | Supports breadth-first search algorithms |
Typically used in scenarios requiring backtracking | Commonly used for tasks with time-sensitive priorities |
Can be implemented using recursion for certain algorithms | Not commonly implemented using recursion due to FIFO nature |
Commonly used for handling function calls and local variables | Commonly used for managing tasks with different priorities |
Efficient for applications with a limited number of elements | Efficient for scenarios requiring access to elements in order of arrival |
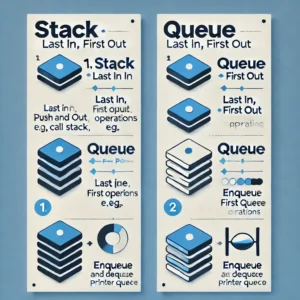
Practical Implementation
Detailed Practical Implementation Examples
Stack Implementation in Python:
class Stack:
def __init__(self):
self.items = []
def is_empty(self):
return len(self.items) == 0
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def peek(self):
if not self.is_empty():
return self.items[-1]
stack = Stack()
stack.push(1)
stack.push(2)
print(stack.pop())
print(stack.peek())
Queue Implementation in Java:
import java.util.LinkedList;import java.util.Queue;
public class MyQueue {
private Queue<Integer> queue = new LinkedList<>();
public void enqueue(int item) {
queue.offer(item);
}
public int dequeue() {
return queue.poll();
}
public int peek() {
return queue.peek();
}
public boolean isEmpty() {
return queue.isEmpty();
}
}
Step-by-Step Implementation Guide
1. Define the data structure class (Stack or Queue).
2. Implement necessary methods like push, pop, peek for Stack, or enqueue, dequeue, peek for Queue.
3. Test the implementation with sample data to ensure correctness.
Best Practices and Optimization Tips
- Use built-in data structures: Whenever possible, use language-provided data structures as they are optimized for performance.
- Keep methods simple: Each method in the data structure class should have a clear and single responsibility.
- Optimize data access: Minimize unnecessary iteration or manipulation of elements in the data structure.
Common Pitfalls and Solutions
- Pitfall: Forgetting to check for empty structure before performing pop or dequeue operations.
- Solution: Always validate if the structure is empty before performing retrieval operations.
- Pitfall: Incorrectly implementing the order of elements in the structure.
- Solution: Ensure the correct order of elements by following the FIFO principle for queues and LIFO principle for stacks.
Frequently Asked Questions
What are stack and queue data structures?
Stack and queue are two fundamental data structures in computer science. A stack follows the Last In, First Out (LIFO) principle, where the last element added is the first one to be removed. On the other hand, a queue adheres to the First In, First Out (FIFO) principle, where the first element added is the first to be removed.
When should I use a stack data structure?
A stack is suitable for scenarios where you need to track function calls, implement undo functionality, or perform depth-first search algorithms. It is efficient for managing function execution order and handling recursive operations.
What are common applications of a queue data structure?
Queues are commonly used in scenarios such as task scheduling, printer spooling, and breadth-first search algorithms. They are effective in managing processes in the order they arrive and ensuring fairness in resource allocation.
How does a stack differ from a queue in terms of operations?
A stack supports two primary operations: push (to insert an element) and pop (to remove the top element). In contrast, a queue supports operations such as enqueue (to add an element to the rear) and dequeue (to remove an element from the front).
Can a stack and a queue be implemented using the same underlying data structure?
Yes, both stack and queue can be implemented using arrays or linked lists. However, the way elements are added and removed differs based on whether it is being used as a stack or a queue. Stacks typically use arrays with push and pop operations, while queues use arrays with enqueue and dequeue operations.
Conclusion
In conclusion, the comparison between stack and queue data structures has revealed significant differences in their functionality and usage. Stacks prioritize Last In First Out (LIFO) ordering, while queues adhere to First In First Out (FIFO) ordering. These distinctions play a crucial role in determining the most suitable choice for specific applications.
Based on the analysis, clear recommendations can be made for decision-making. For scenarios where the order of processing is critical and needs to follow a strict sequence, queues are the preferred data structure. On the other hand, if there is a requirement for efficient access to the most recent data for processing, stacks offer a more suitable solution.
When deciding between stack and queue data structures, key criteria to consider include the specific requirements of the application in terms of data processing order, performance considerations, and ease of implementation. By carefully evaluating these factors, developers can make an informed decision on whether to utilize a stack or a queue based on the unique needs of their project.